C Exercises: Print the alphabet set in decimal and character form
Display alphabet letters with their ASCII codes
Write a C program to print the alphabet set in decimal and character form.
Pictorial Presentation:
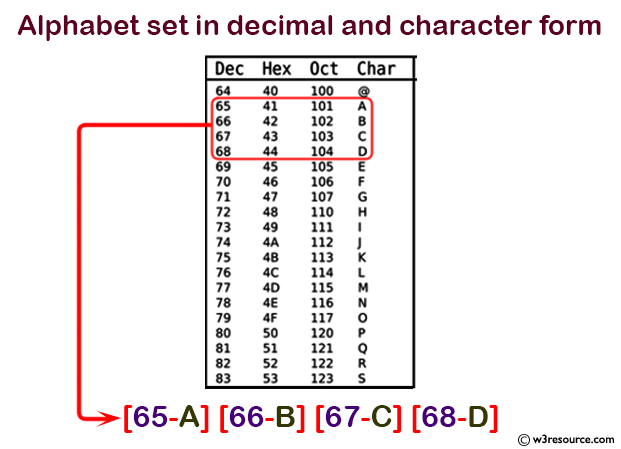
Sample Solution:
C Code:
#include <stdio.h>
#define N 10
int main() {
char chr;
printf("\n");
// Loop through ASCII values from 65 ('A') to 122 ('z')
for (chr = 65; chr <= 122; chr = chr + 1) {
// Exclude characters between 'Z' and 'a'
if (chr > 90 && chr < 97)
continue;
printf("[%2d-%c] ", chr, chr);
}
return 0;
}
Sample Output:
[65-A] [66-B] [67-C] [68-D] [69-E] [70-F] [71-G] [72-H] [73-I] [74-J] [75-K] [76-L] [77-M] [78-N] [79-O] [80-P] [81-Q] [82-R] [83-S] [84-T] [85-U] [86-V] [87-W] [88-X] [89-Y] [90-Z] [97-a] [98-b] [99-c] [100-d] [101-e] [102-f] [103-g] [104-h] [105-i] [106-j] [107-k] [108-l] [109-m] [110-n] [111-o] [112-p] [113-q] [114-r] [115-s] [116-t] [117-u] [118-v] [119-w] [120-x] [121-y] [122-z]
Flowchart:
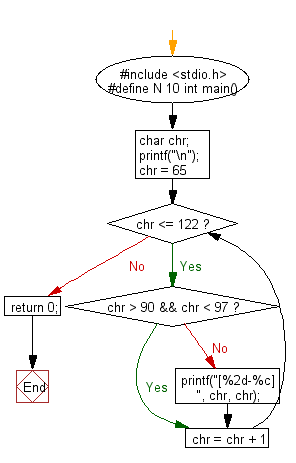
For more Practice: Solve these Related Problems:
- Write a C program to display the alphabet in both uppercase and lowercase with their ASCII values in hexadecimal.
- Write a C program to print the ASCII codes of all letters in reverse order.
- Write a C program to display ASCII values for vowels and consonants separately in a formatted table.
- Write a C program to output the alphabet along with ASCII values and their binary representations.
C programming Code Editor:
Previous:Write a C program to print a binomial coefficient table.
Next: Write a C program to copy a given string into another and count the number of characters copied.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.