C Exercises: Copy a given string into another and count the number of characters copied
Copy a string into another and count its characters
Write a C program to copy a given string into another and count the number of characters copied.
Pictorial Presentation:
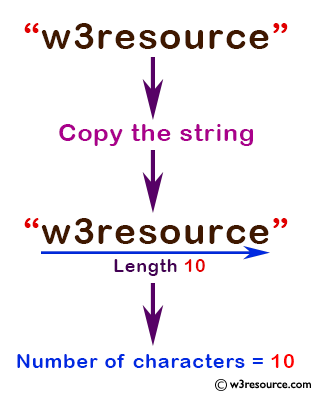
Sample Solution:
C Code:
#include<stdio.h>
#define N 10
int main() {
char str1[80], str2[80];
int i;
// Prompt for user input
printf("Input a string: ");
scanf("%s", str2);
// Copy characters from str2 to str1
for(i=0; str2[i]!='\0'; i++)
str1[i]=str2[i];
str1[i]='\0';
// Output results
printf("\n");
printf("Original string: %s", str1);
printf("\nNumber of characters = %d\n", i);
return 0;
}
Sample Output:
Input a string Original string: w3resource Number of characters = 10
Flowchart:
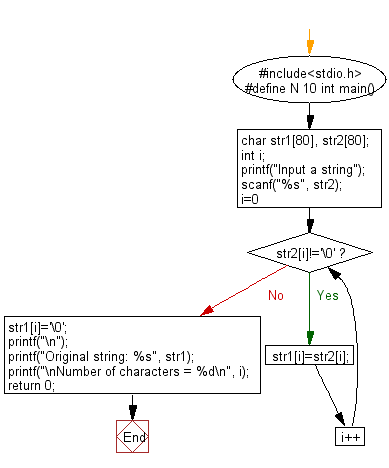
For more Practice: Solve these Related Problems:
- Write a C program to copy a string without using strcpy and count its characters using pointer arithmetic.
- Write a C program to duplicate a string in reverse order and count the characters copied.
- Write a C program to copy a string and convert all lowercase letters to uppercase while counting the characters.
- Write a C program to copy a string and simultaneously count the occurrences of a specified character.
C programming Code Editor:
Previous:Write a C program to print the alphabet set in decimal and character form.
Next: Write a C program to remove any negative sign in front of a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.