C Exercises: Remove any negative sign in front of a number
Remove a negative sign from a number
Write a C program to remove any negative sign in front of a number.
Pictorial Presentation:
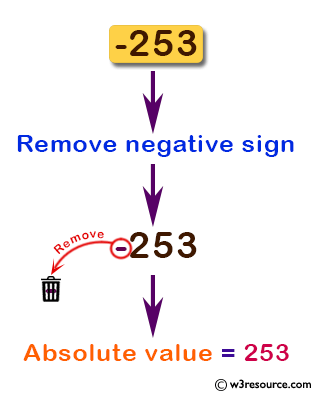
Sample Solution:
C Code:
#include<stdio.h>
#define N 10
// Function prototype declaration
int abs_val(int);
int main() {
int x, result;
// Prompt for user input
printf("Input a value (negative): \n");
scanf("%d", &x);
printf("Original value = %d", x);
// Call the abs_val function and store the result
result = abs_val(x);
// Output the result
printf("\nAbsolute value = %d", result);
return 0;
}
// Function to calculate the absolute value
int abs_val(int y) {
if(y < 0)
return(y * -1);
else
return(y);
}
Sample Output:
Input a value (negative): Original value = -253 Absolute value = 253
Flowchart:
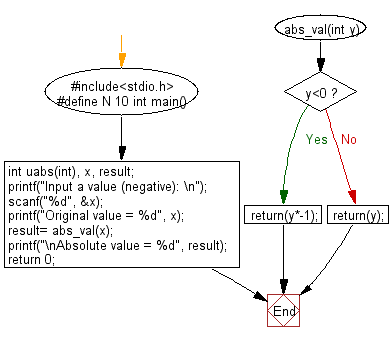
For more Practice: Solve these Related Problems:
- Write a C program to compute the absolute value of a number without using the abs() function.
- Write a C program to remove a negative sign from an integer input using bitwise operations.
- Write a C program to convert a negative floating-point number to its positive equivalent without library functions.
- Write a C program to conditionally remove the negative sign from a number and display a formatted output.
C programming Code Editor:
Previous: Write a C program to print the alphabet set in decimal and character form.
Next: Write a C programming that reads in two integers and check whether the first integer is a multiple of the second integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.