C Exercises: Print a table of all the Roman numeral equivalents of the decimal numbers in the range 1 to 50
Print Roman numeral equivalents for numbers 1 to 50
Write a C program to print a table of all the Roman numeral equivalents of decimal numbers in the range 1 to 50.
Sample Solution:
C Code:
#include <stdio.h>
int main()
{
int i, x;
// Print the header of the Roman numeral table
printf("Decimal Roman\n");
printf("number numeral\n");
printf("-------------------\n");
// Loop through numbers from 1 to 100
for(i = 1; i<= 100; i++)
{
x = i; // Store current value of i in x
printf("%d ", x); // Print the decimal number
// Convert decimal number to Roman numeral
if(x == 100) {
printf("C"); // Print Roman numeral for 100
x = 0; // Reset x to 0
}
if(x >= 50) {
printf("L"); // Print Roman numeral for 50
x -= 50; // Subtract 50 from x
}
while(x >= 10) {
printf("X"); // Print Roman numeral for 10
x -= 10; // Subtract 10 from x
}
if(x >= 5) {
if(x % 10 == 9) {
printf("IX"); // Print Roman numeral for 9
x -= 9; // Subtract 9 from x
}
else {
printf("V"); // Print Roman numeral for 5
x -= 5; // Subtract 5 from x
}
}
while(x > 0)
{
if(x % 10 == 4) {
printf("IV"); // Print Roman numeral for 4
x -= 4; // Subtract 4 from x
}
else {
printf("I"); // Print Roman numeral for 1
x -= 1; // Subtract 1 from x
}
}
printf("\n"); // Move to the next line for the next number
}
return 0;
}
Sample Output:
Decimal Roman numbers numerals ------------------- 1 I 2 II 3 III 4 IV 5 V 6 VI 7 VII 8 VIII 9 IX 10 X 11 XI 12 XII 13 XIII 14 XIV 15 XV 16 XVI 17 XVII 18 XVIII 19 XIX 20 XX 21 XXI 22 XXII 23 XXIII 24 XXIV 25 XXV 26 XXVI 27 XXVII 28 XXVIII 29 XXIX 30 XXX 31 XXXI 32 XXXII 33 XXXIII 34 XXXIV 35 XXXV 36 XXXVI 37 XXXVII 38 XXXVIII 39 XXXIX 40 XL 41 XLI 42 XLII 43 XLIII 44 XLIV 45 XLV 46 XLVI 47 XLVII 48 XLVIII 49 XLIX 50 L 51 LI 52 LII 53 LIII 54 LIV 55 LV 56 LVI 57 LVII 58 LVIII 59 LIX 60 LX 61 LXI 62 LXII 63 LXIII 64 LXIV 65 LXV 66 LXVI 67 LXVII 68 LXVIII 69 LXIX 70 LXX 71 LXXI 72 LXXII 73 LXXIII 74 LXXIV 75 LXXV 76 LXXVI 77 LXXVII 78 LXXVIII 79 LXXIX 80 LXXX 81 LXXXI 82 LXXXII 83 LXXXIII 84 LXXXIV 85 LXXXV 86 LXXXVI 87 LXXXVII 88 LXXXVIII 89 LXXXIX 90 XC 91 XCI 92 XCII 93 XCIII 94 XCIV 95 XCV 96 XCVI 97 XCVII 98 XCVIII 99 XCIX 100 C
Flowchart:
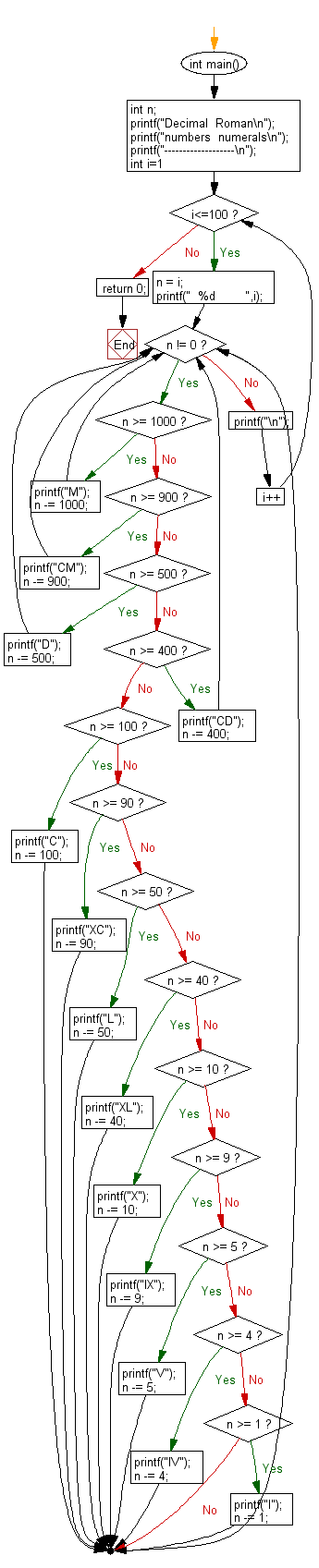
For more Practice: Solve these Related Problems:
- Write a C program to convert numbers from 1 to 50 into their Roman numeral representations using arrays.
- Write a C program to generate a table of numbers and their corresponding Roman numerals with error handling.
- Write a C program to convert user-specified numbers into Roman numerals within the range 1 to 50.
- Write a C program to display Roman numeral equivalents for numbers 1 to 50 using a switch-case construct.
C programming Code Editor:
Previous:Write a C program to calculate and print the average of some integers. Accept all the values preceding 888.
Next: Write a C program to display the sizes and ranges for each of C's data types.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.