Display the sizes and ranges for each of C's data types
Display sizes and ranges of C's data types
Write a C program to display the sizes and ranges for each of C's data types.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdint.h>
#include <stdbool.h>
#include <limits.h>
int main(void) {
// Display title
printf("Size and Range of C data types:\n\n");
// Display column headers
printf("%-20s %-20s %-20s\n", "Type", "Bytes", "Range");
// Display separator line
printf("------------------------------------------------------\n");
// Print size and range of various data types
printf("%-20s %lu %-20d to %-20d\n", "char", sizeof(char), CHAR_MIN, CHAR_MAX);
printf("%-20s %lu %-20d to %-20d\n", "int8_t", sizeof(int8_t), INT8_MIN, INT8_MAX);
printf("%-20s %lu %-20d to %-20d\n", "unsigned char", sizeof(unsigned char), 0, UCHAR_MAX);
printf("%-20s %lu %-20d to %-20d\n", "uint8_t", sizeof(uint8_t), 0, UINT8_MAX);
printf("%-20s %lu %-20d to %-20d\n", "short", sizeof(short), SHRT_MIN, SHRT_MAX);
printf("%-20s %lu %-20d to %-20d\n", "int16_t", sizeof(int16_t), INT16_MIN, INT16_MAX);
printf("%-20s %lu %-20d to %-20d\n", "uint16_t", sizeof(uint16_t), 0, UINT16_MAX);
printf("%-20s %lu %-20d to %-20d\n", "int", sizeof(int), INT_MIN, INT_MAX);
printf("%-20s %lu %-20u to %-20u\n", "unsigned", sizeof(unsigned), 0, UINT_MAX);
printf("%-20s %lu %-20ld to %-20ld\n", "long", sizeof(long), LONG_MIN, LONG_MAX);
printf("%-20s %lu %-20lu to %-20lu\n", "unsigned long", sizeof(unsigned long), 0, ULONG_MAX);
printf("%-20s %lu %-20d to %-20d\n", "int32_t", sizeof(int32_t), INT32_MIN, INT32_MAX);
printf("%-20s %lu %-20u to %-20u\n", "uint32_t", sizeof(uint32_t), 0, UINT32_MAX);
printf("%-20s %lu %-20lld to %-20lld\n", "long long", sizeof(long long), LLONG_MIN, LLONG_MAX);
printf("%-20s %lu %-20llu to %-20llu\n", "int64_t", sizeof(int64_t), 0LL, ULLONG_MAX);
printf("%-20s %lu %-20lld to %-20lld\n", "int64_t", sizeof(int64_t), LLONG_MIN, LLONG_MAX);
printf("%-20s %lu %-20llu to %-20llu\n", "uint64_t", sizeof(uint64_t), 0ULL, ULLONG_MAX);
// Floating point types don't have well-defined "range" in the same sense as integers
// Add a newline for better output formatting
printf("\n");
// Indicate successful execution of the program
return 0;
}
Sample Output:
Size and Range of C data types: Type Bytes Range ------------------------------------------------------ char 1 -128 to 127 int8_t 1 -128 to 127 unsigned char 1 0 to 255 uint8_t 1 0 to 255 short 2 -32768 to 32767 int16_t 2 -32768 to 32767 uint16_t 2 0 to 65535 int 4 -2147483648 to 2147483647 unsigned 4 0 to 4294967295 long 4 -2147483648 to 2147483647 unsigned long 4 0 to 4294967295 int32_t 4 -2147483648 to 2147483647 uint32_t 4 0 to 4294967295 long long 8 -9223372036854775808 to 9223372036854775807 int64_t 8 0 to 18446744073709551615 int64_t 8 -9223372036854775808 to 9223372036854775807 uint64_t 8 0 to 18446744073709551615
Flowchart:
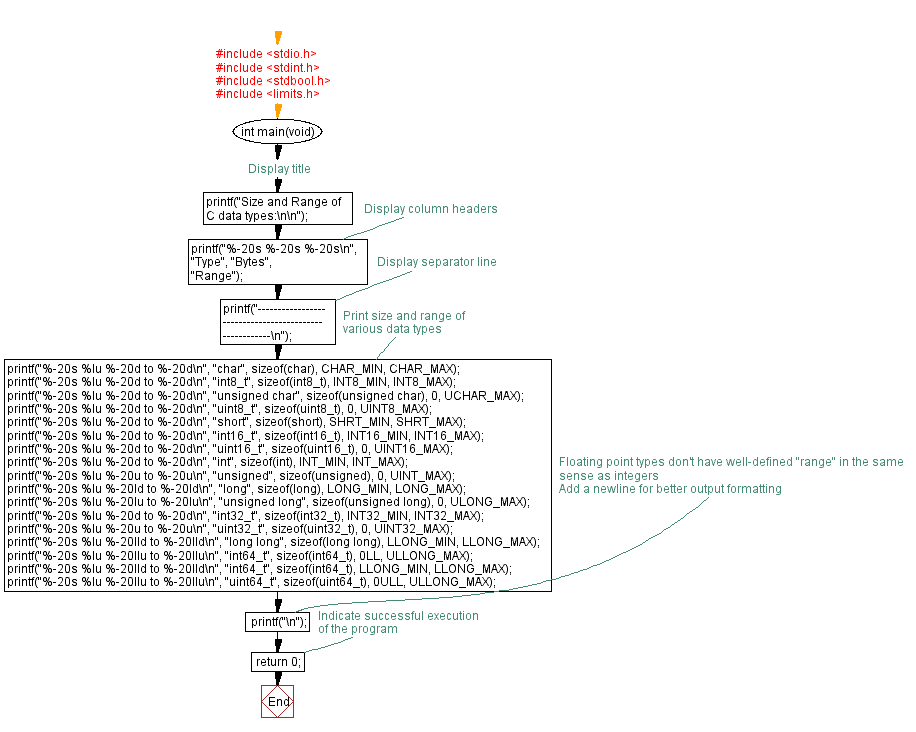
For more Practice: Solve these Related Problems:
- Write a C program to display the size and range of basic data types using sizeof and limits.h.
- Write a C program to print sizes of various data types and their minimum and maximum values with formatted output.
- Write a C program to display the sizes and ranges of signed and unsigned data types using conditional compilation.
- Write a C program to compute and display the sizes and ranges of standard C data types, including custom typedefs.
C programming Code Editor:
Previous:Write a C program to print a table of all the Roman numeral equivalents of the decimal numbers in the range 1 to 50.
Next:Write a C program to display the minimum and maximum values for each of C's data types.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.