C Exercises: String representation of common directory paths
16. Common Directory Tree
A set of strings represent directory paths and a single character directory separator (/).
Write a program in C language to get a part of the directory tree that is common to all directories.
Test Data:
({/home/me/user/temp/a", "/home/me/user/temp/b","/home/me/user/temp/c/d"}) ->
/home/me/user/temp
Sample Solution:
C Code:
#include <stdio.h>
int common_path(const char *const *names, int n, char sep)
{
int i, pos_len;
for (pos_len = 0; ; pos_len++) {
for (i = 0; i < n; i++) {
if (names[i][pos_len] != '\0' &&
names[i][pos_len] == names[0][pos_len])
continue;
while (pos_len > 0 && names[0][--pos_len] != sep);
return pos_len;
}
}
return 0;
}
int main()
{
const char *names[] = {
"/home/me/user/temp/a",
"/home/me/user/temp/b",
"/home/me/user/temp/c/d",
};
int len = common_path(names, sizeof(names) / sizeof(const char*), '/');
if (!len)
printf("No common path\n");
else
printf("Common path: %.*s\n", len, names[0]);
return 0;
}
Sample Output:
Common path: /home/me/user/temp
Flowchart:
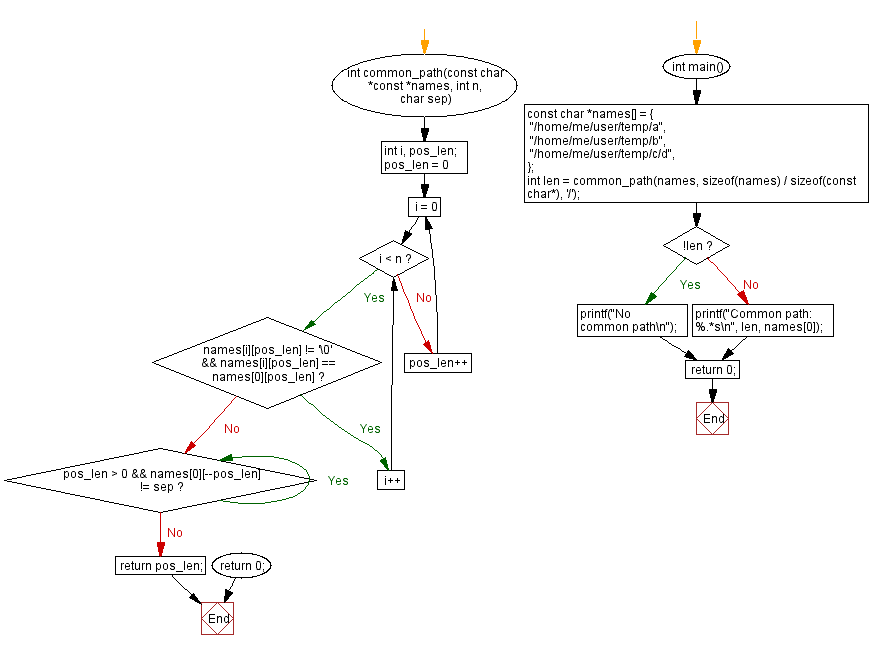
For more Practice: Solve these Related Problems:
- Write a C program to compare multiple directory paths and output the longest common prefix.
- Write a C program to extract the common subdirectory from a list of file paths with different separators.
- Write a C program to parse directory paths and display the directory tree structure that is shared by all paths.
- Write a C program to find the common root directory from a list of absolute paths and print it in normalized form.
C Programming Code Editor:
Previous: Remove a file from the disk.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.