C Exercises: Read an existing file
2. Read Existing File
Write a program in C to read an existing file.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
void main()
{
FILE *fptr;
char fname[20];
char str;
printf("\n\n Read an existing file :\n");
printf("------------------------------\n");
printf(" Input the filename to be opened : ");
scanf("%s",fname);
fptr = fopen (fname, "r");
if (fptr == NULL)
{
printf(" File does not exist or cannot be opened.\n");
exit(0);
}
printf("\n The content of the file %s is :\n",fname);
str = fgetc(fptr);
while (str != EOF)
{
printf ("%c", str);
str = fgetc(fptr);
}
fclose(fptr);
printf("\n\n");
}
Sample Output:
Read and existing file : ------------------------------ Input the filename to be opened : test.txt The content of the file test.txt is : This is the content of the file test.txt
Flowchart:
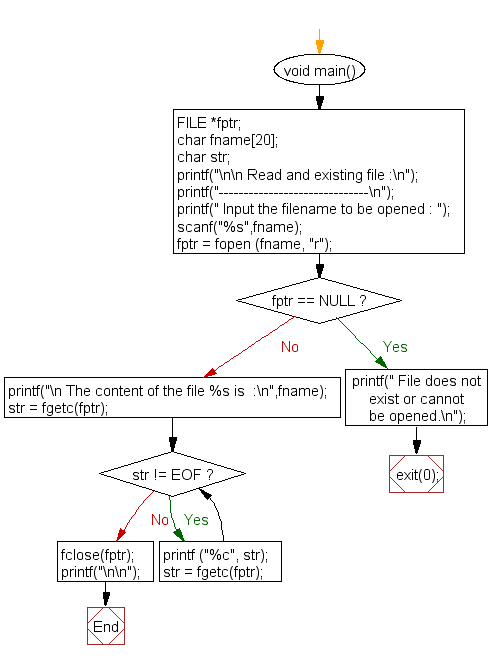
For more Practice: Solve these Related Problems:
- Write a C program to read a text file and display its content in reverse order (line by line).
- Write a C program to read a file and count the occurrence of a specific keyword provided by the user.
- Write a C program to read a file and print only the lines that contain a given substring.
- Write a C program to read a file and output its content to the console along with the line numbers.
C Programming Code Editor:
Previous: Write a program in C to create and store information in a text file.
Next: Write a program in C to write multiple lines in a text file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.