C Exercises: Write multiple lines in a text file and read the file
C File Handling : Exercise-3 with Solution
Write a program in C to write multiple lines to a text file.
Sample Solution:
C Code:
#include <stdio.h>
int main ()
{
FILE * fptr;
int i,n;
char str[100];
char fname[20]="test.txt";
char str1;
printf("\n\n Write multiple lines in a text file and read the file :\n");
printf("------------------------------------------------------------\n");
printf(" Input the number of lines to be written : ");
scanf("%d", &n);
printf("\n :: The lines are ::\n");
fptr = fopen (fname,"w");
for(i = 0; i < n+1;i++)
{
fgets(str, sizeof str, stdin);
fputs(str, fptr);
}
fclose (fptr);
/*-------------- read the file -------------------------------------*/
fptr = fopen (fname, "r");
printf("\n The content of the file %s is :\n",fname);
str1 = fgetc(fptr);
while (str1 != EOF)
{
printf ("%c", str1);
str1 = fgetc(fptr);
}
printf("\n\n");
fclose (fptr);
return 0;
}
Sample Output:
Write multiple lines in a text file and read the file : ------------------------------------------------------------ Input the number of lines to be written : 4 :: The lines are :: test line 1 test line 2 test line 3 test line 4 The content of the file test.txt is : test line 1 test line 2 test line 3 test line 4
Flowchart:
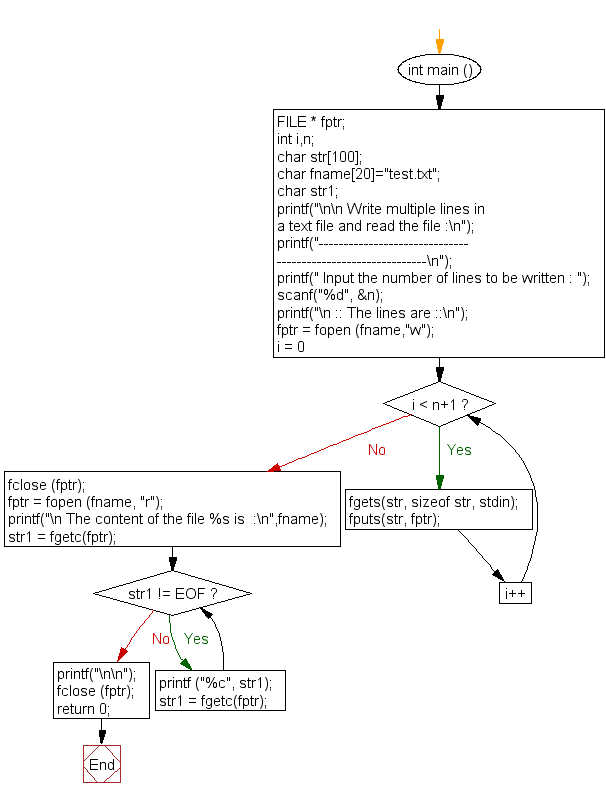
C Programming Code Editor:
Previous: Write a program in C to read an existing file.
Next: Write a program in C to read the file and store the lines into an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics