C Exercises: Replace a specific line in a file with a new text
9. Replace Specific Line in File
Write a program in C to replace a specific line with another text in a file.
Assume that the content of the file test.txt is : test line 1 test line 2 test line 3 test line 4
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
#define MAX 256
int main()
{
FILE *fptr1, *fptr2;
int lno, linectr = 0;
char str[MAX],fname[MAX];
char newln[MAX], temp[] = "temp.txt";
printf("\n\n Replace a specific line in a text file with a new text :\n");
printf("-------------------------------------------------------------\n");
printf(" Input the file name to be opened : ");
fgets(fname, MAX, stdin);
fname[strlen(fname) - 1] = '\0';
fptr1 = fopen(fname, "r");
if (!fptr1)
{
printf("Unable to open the input file!!\n");
return 0;
}
fptr2 = fopen(temp, "w");
if (!fptr2)
{
printf("Unable to open a temporary file to write!!\n");
fclose(fptr1);
return 0;
}
/* get the new line from the user */
printf(" Input the content of the new line : ");
fgets(newln, MAX, stdin);
/* get the line number to delete the specific line */
printf(" Input the line no you want to replace : ");
scanf("%d", &lno);
lno++;
// copy all contents to the temporary file other except specific line
while (!feof(fptr1))
{
strcpy(str, "\0");
fgets(str, MAX, fptr1);
if (!feof(fptr1))
{
linectr++;
if (linectr != lno)
{
fprintf(fptr2, "%s", str);
}
else
{
fprintf(fptr2, "%s", newln);
}
}
}
fclose(fptr1);
fclose(fptr2);
remove(fname);
rename(temp, fname);
printf(" Replacement did successfully..!! \n");
return 0;
}
Sample Output:
Replace a specific line in a text file with a new text : ------------------------------------------------------------- Input the file name to be opened : test.txt Input the content of the new line : 2 Input the line no you want to replace : 2 Replacement did successfully..!!
Flowchart:
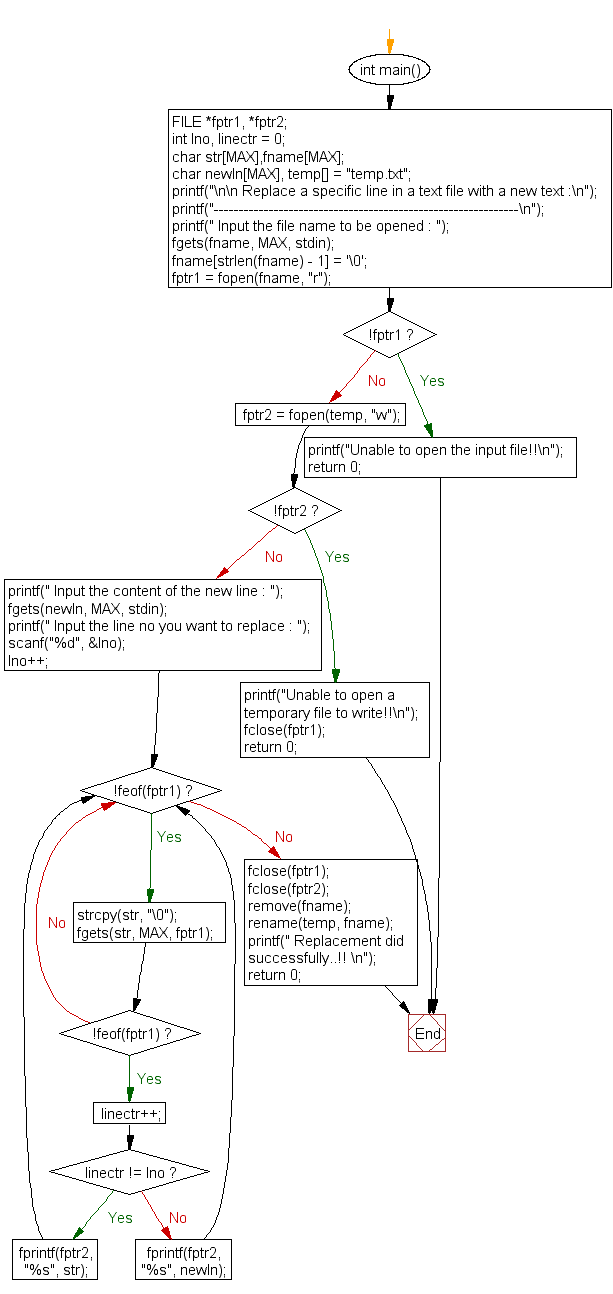
For more Practice: Solve these Related Problems:
- Write a C program to replace all occurrences of a specific word in a file with another word.
- Write a C program to replace a particular line in a file based on a search string, not just by line number.
- Write a C program to update a file by replacing the content of a specified line with user input and then display the modified file.
- Write a C program to replace multiple lines in a file by mapping each old line to a new line using a configuration file.
C Programming Code Editor:
Previous: Write a program in C to delete a specific line from a file.
Next: Write a program in C to append multiple lines at the end of a text file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.