C Exercises: Delete a specific line from a file
C File Handling : Exercise-8 with Solution
Write a program in C to delete a specific line from a file.
Assume that the content of the file test.txt is : test line 1 test line 2 test line 3 test line 4
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
#define MAX 256
int main()
{
int lno, ctr = 0;
char ch;
FILE *fptr1, *fptr2;
char fname[MAX];
char str[MAX], temp[] = "temp.txt";
printf("\n\n Delete a specific line from a file :\n");
printf("-----------------------------------------\n");
printf(" Input the file name to be opened : ");
scanf("%s",fname);
fptr1 = fopen(fname, "r");
if (!fptr1)
{
printf(" File not found or unable to open the input file!!\n");
return 0;
}
fptr2 = fopen(temp, "w"); // open the temporary file in write mode
if (!fptr2)
{
printf("Unable to open a temporary file to write!!\n");
fclose(fptr1);
return 0;
}
printf(" Input the line you want to remove : ");
scanf("%d", &lno);
lno++;
// copy all contents to the temporary file except the specific line
while (!feof(fptr1))
{
strcpy(str, "\0");
fgets(str, MAX, fptr1);
if (!feof(fptr1))
{
ctr++;
/* skip the line at given line number */
if (ctr != lno)
{
fprintf(fptr2, "%s", str);
}
}
}
fclose(fptr1);
fclose(fptr2);
remove(fname); // remove the original file
rename(temp, fname); // rename the temporary file to original name
/*------ Read the file ----------------*/
fptr1=fopen(fname,"r");
ch=fgetc(fptr1);
printf(" Now the content of the file %s is : \n",fname);
while(ch!=EOF)
{
printf("%c",ch);
ch=fgetc(fptr1);
}
fclose(fptr1);
/*------- End of reading ---------------*/
return 0;
}
Sample Output:
Delete a specific line from a file : ----------------------------------------- Input the file name to be opened : test.txt Input the line you want to remove : 2 Now the content of the file test.txt are : test line 1 test line 3 test line 4
Flowchart:
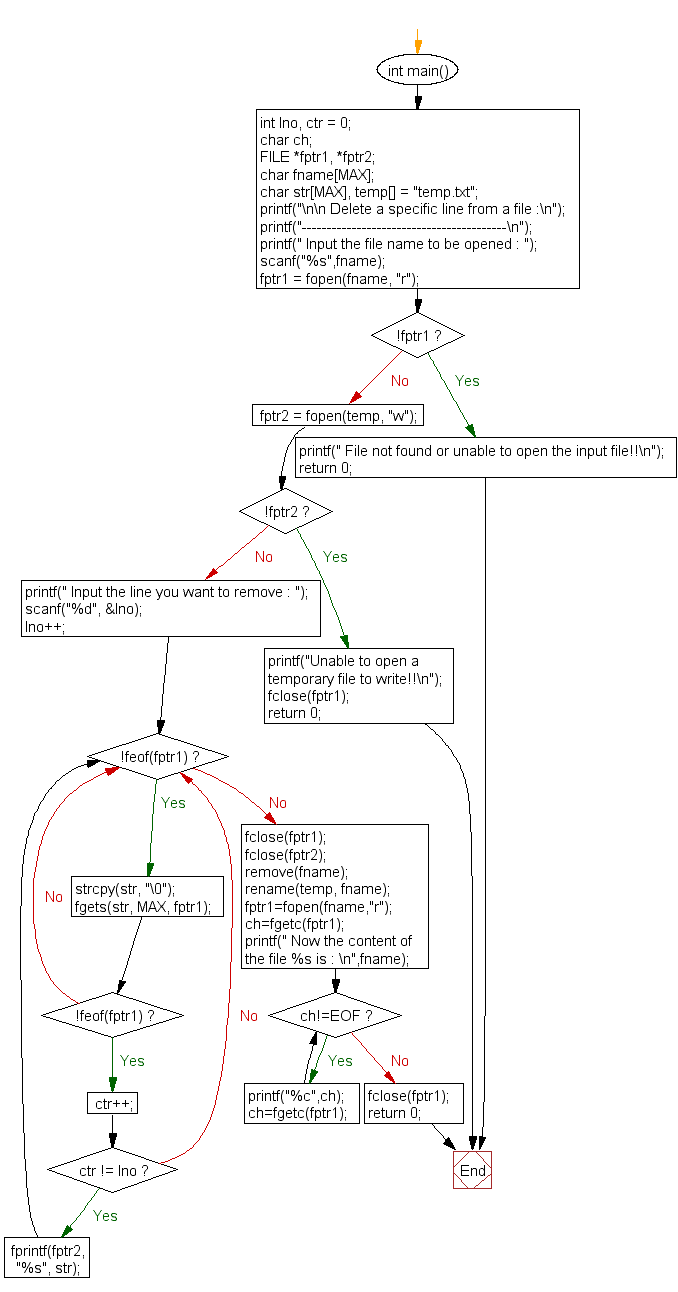
C Programming Code Editor:
Previous: Write a program in C to count number of words and characters in a file.
Next: Write a program in C to replace a specific line with another text in a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/file-handling/c-file-handling-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics