C Exercises: Calculate the factorial of a given number
15. Factorial Calculation
Write a C program to calculate the factorial of a given number.
Step-by-step explanation of how a typical C program calculates the factorial of a given number:
- Include Standard Libraries: The program starts by including the standard input-output library (stdio.h) which allows the program to use functions like "printf()" and "scanf()".
- Main Function: The "main()" function is the entry point of the program where execution begins.
- Declare Variables: The program declares an integer variable, typically n, to store the number for which the factorial is to be calculated. Another variable, often called 'result', is initialized to 1 to store the factorial result.
- Input the Number: The program prompts the user to enter a number and reads the input using the "scanf()" function. This input is stored in the variable 'n'.
- Factorial Calculation (Using a Loop):
- A loop (such as a "for" loop or "while" loop) runs from 1 to the given number 'n'.
- In each iteration of the loop, the current value of the loop counter is multiplied by 'result', and the result is stored back in 'result'.
- For example, if n is 5, the loop iterates as follows:
- result = result * 1 (result is now 1)
- result = result * 2 (result is now 2)
- result = result * 3 (result is now 6)
- result = result * 4 (result is now 24)
- result = result * 5 (result is now 120)
- Output the Result: After the loop completes, the program prints the value of 'result', which now holds the factorial of the input number 'n'.
- Return Statement: The "main()" function returns 0 to indicate that the program executed successfully.
Visual Presentation:
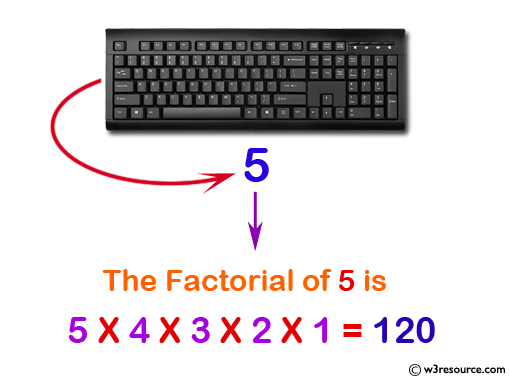
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main(){
int i, f = 1, num; // Declare variables 'i' for loop counter, 'f' to store factorial, 'num' for user input.
printf("Input the number : "); // Print a message to prompt user input.
scanf("%d", &num); // Read the value of 'num' from the user.
for(i = 1; i <= num; i++) // Start a loop to calculate factorial.
f = f * i; // Calculate factorial.
printf("The Factorial of %d is: %d\n", num, f); // Print the calculated factorial.
}
Output:
Input the number : 5 The Factorial of 5 is: 120
Explanation:
for (i = 1; i <= num; i++) f = f * i;
In the above for loop, the variable i is initialized to 1, and the loop will continue as long as i is less than or equal to the value of variable 'num'. In each iteration of the loop, the value of variable 'f' is updated by multiplying it with the value of i using the assignment operator (*=).
The loop will increment the value of i by 1, and the process will repeat until the condition i<=num is no longer true.
Flowchart:
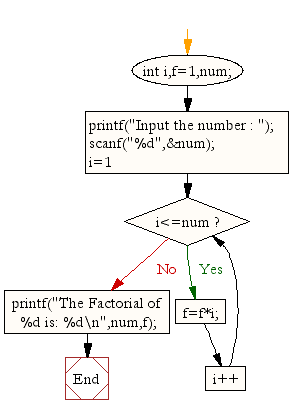
C Programming Code Editor:
Previous: Write a program in C to make such a pattern like a pyramid with an asterisk.
Next: Write a program in C to display the n terms of even natural number and their sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.