C Exercises: Calculate n terms of even natural number and their sum
C For Loop: Exercise-16 with Solution
Write a C program to display the sum of n terms of even natural numbers.
This C program calculates and displays the sum of the first n even natural numbers. By iterating through even numbers up to the specified limit, the program accumulates their sum, demonstrating the use of loops and arithmetic operations in C. This task highlights the ability to handle sequences and perform basic summation tasks programmatically.
Visual Presentation:
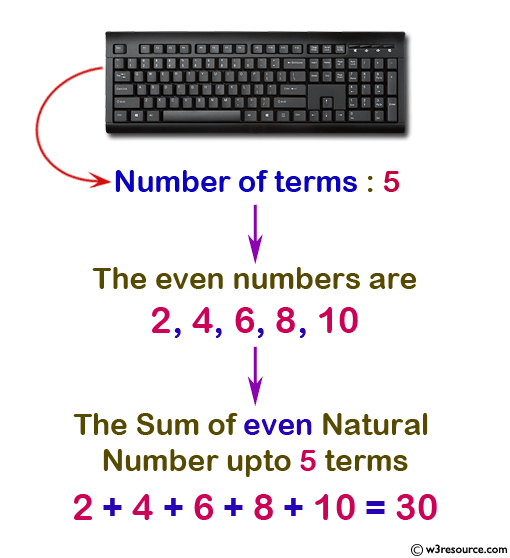
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, n, sum = 0; // Declare variables 'i' for loop counter, 'n' for user input, 'sum' to store the sum.
printf("Input number of terms : "); // Prompt the user to input the number of terms.
scanf("%d", &n); // Read the value of 'n' from the user.
printf("\nThe even numbers are :"); // Print a message.
for(i = 1; i <= n; i++) // Start a loop to generate even numbers.
{
printf("%d ", 2 * i); // Print the even number.
sum += 2 * i; // Add the even number to the sum.
}
printf("\nThe Sum of even Natural Number upto %d terms : %d \n", n, sum); // Print the sum of even numbers.
}
Output:
Input number of terms : 5 The even numbers are :2 4 6 8 10 The Sum of even Natural Number upto 5 terms : 30
Flowchart:
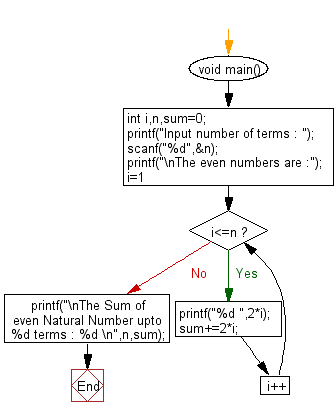
C Programming Code Editor:
Previous: Write a C program to calculate the factorial of a given number.
Next: Write a program in C to make such a pattern like a pyramid with a number which will repeat the number in the same row.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics