C Exercises: Display the pattern like a pyramid with a number which will repeat the number in the same row
17. Pyramid Pattern with Repeated Numbers
Write a C program to make such a pattern like a pyramid with a number which will repeat the number in the same row.
The pattern is as follows:
1 2 2 3 3 3 4 4 4 4
This C program generates a pyramid pattern where each row contains repeated numbers corresponding to the row number. For example, the first row displays '1', the second row displays '2 2', and so on, up to the specified number of rows. The program uses nested loops to create the pyramid structure, with careful formatting to align the numbers correctly.
Visual Presentation:
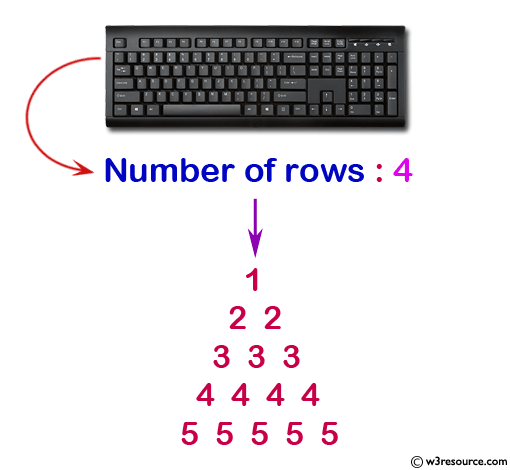
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, j, spc, rows, k; // Declare variables 'i', 'j' for loop counters, 'spc' for spaces, 'rows' for user input, 'k' for loop counter.
printf("Input number of rows : "); // Prompt the user to input the number of rows.
scanf("%d", &rows); // Read the value of 'rows' from the user.
spc = rows + 4 - 1; // Calculate the initial number of spaces.
for(i = 1; i <= rows; i++) // Loop through each row.
{
for(k = spc; k >= 1; k--) // Loop to print spaces.
{
printf(" ");
}
for(j = 1; j <= i; j++) // Loop to print numbers.
{
printf("%d ", i);
}
printf("\n"); // Move to the next line.
spc--; // Decrease the number of spaces for the next row.
}
}
Output:
Input number of rows : 5 1 2 2 3 3 3 4 4 4 4 5 5 5 5 5
This C program generates a pyramid pattern where each row contains repeated numbers corresponding to the row number. For example, the first row displays '1', the second row displays '2 2', and so on, up to the specified number of rows. The program uses nested loops to create the pyramid structure, with careful formatting to align the numbers correctly.
Flowchart:
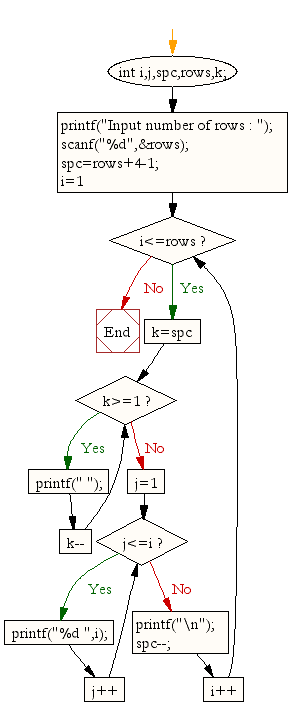
For more Practice: Solve these Related Problems:
- Write a C program to display a pyramid where each row repeats its row number and then print the sum of each row.
- Write a C program to display a pyramid pattern with repeated numbers, alternating the order for even-numbered rows.
- Write a C program to generate a pyramid of repeated numbers using recursion.
- Write a C program to print a pyramid of repeated numbers and calculate the total sum of all numbers in the pyramid.
C Programming Code Editor:
Previous: Write a program in C to display the n terms of even natural number and their sum.
Next: Write a program in C to find the sum of the series [ 1-X^2/2!+X^4/4!- .........].
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.