C Exercises: Display the pattern like a pyramid containing odd number of asterisks
20. Pyramid Pattern with Odd Asterisks
Write a C program to display the pattern as a pyramid using asterisks, with each row containing an odd number of asterisks.
The pattern is as below:
* *** *****
This C program generates a pyramid pattern using asterisks, with each row containing an odd number of asterisks centered to form a pyramid shape. The program prompts the user to input the number of rows and then uses nested loops to print spaces and asterisks in the required pattern.
Visual Presentation:
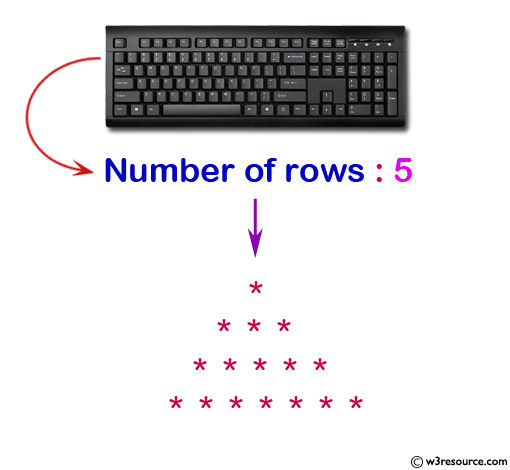
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, j, n; // Declare variables to store input and control loop indices.
// Prompt the user to input the number of rows for the pattern.
printf("Input number of rows for this pattern :");
scanf("%d", &n); // Read the value of 'n' from the user.
for (i = 0; i < n; i++) // Loop for the number of rows.
{
for (j = 1; j <= n - i; j++) // Loop to print spaces before the stars.
printf(" ");
for (j = 1; j <= 2 * i - 1; j++) // Loop to print the stars.
printf("*");
printf("\n"); // Move to the next line after printing each row.
}
}
Output:
Input number of rows for this pattern :5 * *** ***** *******
Flowchart
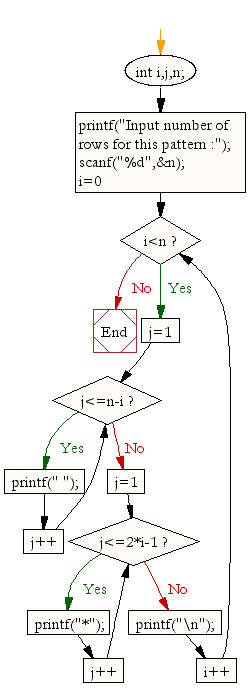
For more Practice: Solve these Related Problems:
- Write a C program to display a centered pyramid where each row contains an odd number of asterisks.
- Write a C program to display an inverted pyramid with odd-numbered asterisk counts.
- Write a C program to print a pyramid of asterisks and then compute the total number of asterisks used.
- Write a C program to display a pyramid of asterisks and then mirror the pattern vertically.
C Programming Code Editor:
Previous: Write a program in C to display the n terms of harmonic series and their sum.
Next: Write a program in C to display the sum of the series [ 9 + 99 + 999 + 9999 ...].
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.