C Exercises: Calculate n terms of square natural number and their sum
C For Loop: Exercise-25 with Solution
Write a C program that displays the n terms of square natural numbers and their sum.
The series is as below:
1 4 9 16 ... n Terms
This C program calculates and displays the first n terms of square natural numbers and their sum. The user inputs the value of nnn, and the program then computes each square. It also calculates the sum of these squares and prints both the individual squares and the total sum.
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, n, sum = 0; // Declare variables for loop control and sum calculation.
printf("Input the number of terms : "); // Prompt the user for input.
scanf("%d", &n); // Read the value of 'n' from the user.
printf("\nThe square natural numbers up to %d terms are :", n); // Print a message.
for(i = 1; i <= n; i++) // Loop to calculate square of natural numbers.
{
printf("%d ", i*i); // Print the square of the current natural number.
sum += i*i; // Add the square to the running sum.
}
printf("\nThe Sum of Square Natural Numbers up to %d terms = %d \n", n, sum); // Print the sum.
}
Output:
Input the number of terms : 5 The square natural upto 5 terms are :1 4 9 16 25 The Sum of Square Natural Number upto 5 terms = 55
Flowchart:
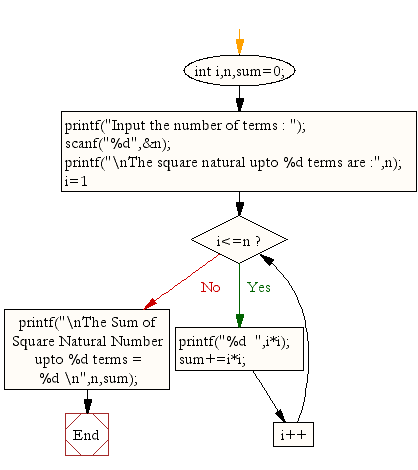
C Programming Code Editor:
Previous: Write a program in C to find the sum of the series [ x - x^3 + x^5 + ......].
Next: Write a program in C to find the sum of the series 1 +11 + 111 + 1111 + .. n terms.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics