C Exercises: Calculate the sum of the series 1 +11 + 111 + 1111 + .. n terms
C For Loop: Exercise-26 with Solution
Write a program in C to find the sum of the series 1 +11 + 111 + 1111 + .. n terms.
This C program calculates the sum of a series where each term is a number composed of repeated digits '1' (i.e., 1, 11, 111, 1111, etc.). The user inputs the number of terms n, and the program then computes and sums these terms, displaying the result. This involves generating each term based on its position and summing them up sequentially.
Visual Presentation:
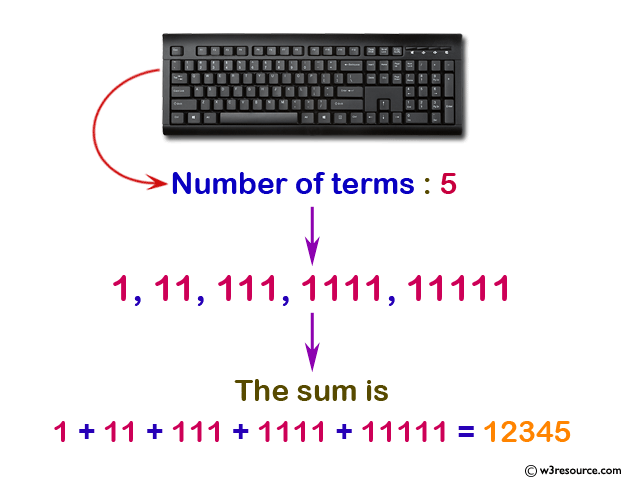
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int n, i; // Declare variables for loop control and input.
long sum = 0; // Initialize a variable to hold the sum of the series.
long int t = 1; // Initialize a variable to generate the series.
printf("Input the number of terms : "); // Prompt the user for input.
scanf("%d", &n); // Read the value of 'n' from the user.
for(i = 1; i <= n; i++) // Loop to generate and sum the series.
{
printf("%ld ", t); // Print the current term of the series.
if (i < n)
{
printf("+ "); // Print addition sign between terms (except the last term).
}
sum = sum + t; // Add the current term to the running sum.
t = (t * 10) + 1; // Generate the next term of the series.
}
printf("\nThe Sum is : %ld\n", sum); // Print the final sum of the series.
}
Output:
Input the number of terms : 5 1 + 11 + 111 + 1111 + 11111 The Sum is : 12345
Flowchart:
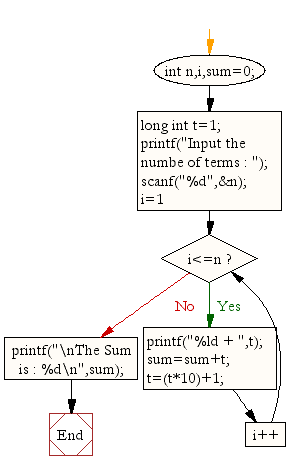
C Programming Code Editor:
Previous: Write a program in C to display the n terms of square natural number and their sum.
Next: Write a c program to check whether a given number is a perfect number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics