C Exercises: Find perfect numbers within a given number of range
C For Loop: Exercise-28 with Solution
Write a C program to find the 'Perfect' numbers within a given number of ranges.
This C program identifies and displays all "Perfect" numbers within a specified range. A perfect number is a positive integer that is equal to the sum of its proper divisors (excluding itself). The user inputs the range limits, and the program iterates through this range to find and print all perfect numbers within it.
Sample Solution:
C Code:
/*
Perfect number is a positive number which sum of all positive divisors excluding
that number is equal to that number. For example 6 is perfect number since divisor of 6 are 1, 2 and 3.
Sum of its divisor is 1 + 2 + 3 = 6
*/
#include <stdio.h> // Include the standard input/output header file.
void main(){
int n, i, sum; // Declare variables for the number being checked, loop control, and sum.
int mn, mx; // Variables for the starting and ending range of numbers.
printf("Input the starting range or number : "); // Prompt the user for the starting range.
scanf("%d", &mn); // Read the starting range from the user.
printf("Input the ending range of number : "); // Prompt the user for the ending range.
scanf("%d", &mx); // Read the ending range from the user.
printf("The Perfect numbers within the given range : "); // Print a message indicating perfect numbers are being displayed.
for(n = mn; n <= mx; n++){ // Loop through the numbers in the specified range.
i = 1; // Initialize the divisor.
sum = 0; // Initialize the sum of divisors.
while(i < n){ // While the divisor is less than the number being checked.
if(n % i == 0) // If 'i' is a divisor of 'n'.
sum = sum + i; // Add 'i' to the sum.
i++; // Increment the divisor.
}
if(sum == n) // If the sum of divisors is equal to the original number.
printf("%d ", n); // Print the perfect number.
}
printf("\n"); // Print a new line for better formatting.
}
Output:
Input the starting range or number : 1 Input the ending range of number : 50 The Perfect numbers within the given range : 6 28
Flowchart:
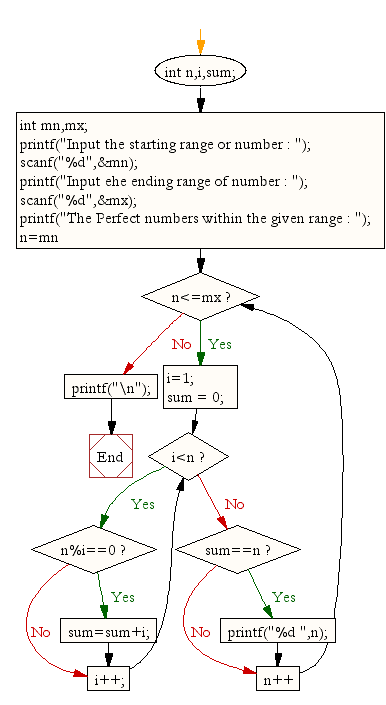
C Programming Code Editor:
Previous: Write a c program to check whether a given number is a perfect number or not.
Next: Write a C program to check whether a given number is an armstrong number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics