C Exercises: Display the pattern like a diamond
31. Diamond Pattern Display
Write a program in C to display a pattern like a diamond.
The pattern is as follows :
* *** ***** ******* ********* ******* ***** *** *
The program should take an integer input representing the number of rows for the top half of the diamond and then print the corresponding pattern. The diamond consists of an upper triangle and an inverted lower triangle, with each row appropriately spaced to maintain the diamond shape.
Visual Presentation:
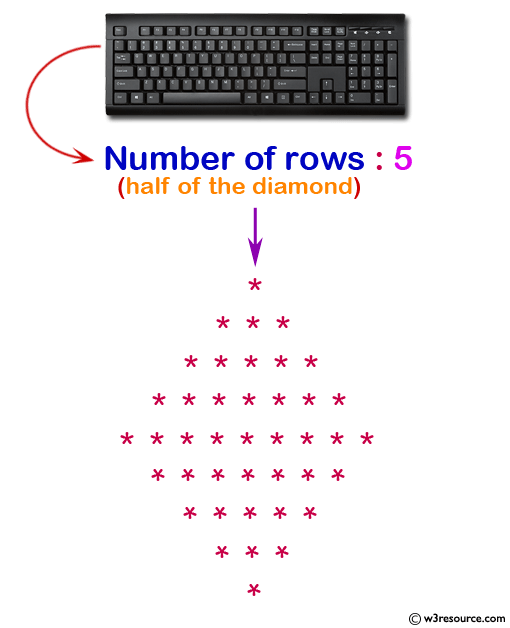
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, j, r; // Declare variables for loop control and the number of rows.
printf("Input number of rows (half of the diamond) :"); // Prompt the user to input the number of rows.
scanf("%d", &r); // Read the number of rows from the user.
for(i = 0; i <= r; i++) // Start a loop to print the upper half of the diamond.
{
for(j = 1; j <= r - i; j++) // Loop to print spaces before the asterisks.
printf(" ");
for(j = 1; j <= 2 * i - 1; j++) // Loop to print asterisks.
printf("*");
printf("\n"); // Move to the next line after completing a row.
}
for(i = r - 1; i >= 1; i--) // Start a loop to print the lower half of the diamond.
{
for(j = 1; j <= r - i; j++) // Loop to print spaces before the asterisks.
printf(" ");
for(j = 1; j <= 2 * i - 1; j++) // Loop to print asterisks.
printf("*");
printf("\n"); // Move to the next line after completing a row.
}
}
Output:
Input number of rows (half of the diamond) :5 * *** ***** ******* ********* ******* ***** *** *
Flowchart:
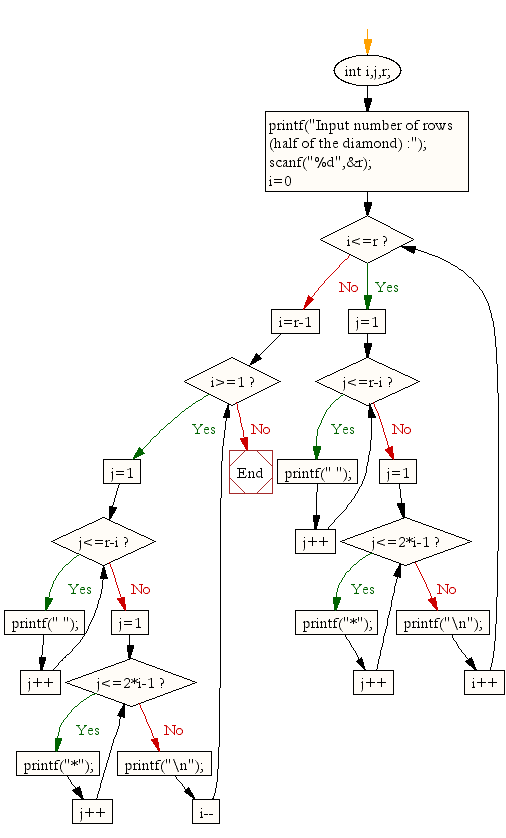
For more Practice: Solve these Related Problems:
- Write a C program to display a diamond pattern using asterisks and then mirror it vertically.
- Write a C program to print a diamond pattern with numbers where each row’s number equals the row index.
- Write a C program to display a diamond pattern with alternating characters for the top and bottom halves.
- Write a C program to print a diamond pattern and then count the total number of asterisks used.
C Programming Code Editor:
Previous: Write a C program to find the Armstrong number for a given range of number.
Next: Write a C program to determine whether a given number is prime or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.