C Exercises: Display the pattern in which the first and a last number of each row will be 1
36. Pattern with 1 as First and Last Number
Write a C program to display a such a pattern for n rows using a number that starts with 1 and each row will have a 1 as the first and last number.
The pattern is as follows:
1 121 12321
Create a C program to display a pyramid pattern with n rows where each row starts and ends with the number 1. The numbers increase sequentially towards the middle of each row and then decrease back to 1. The program should prompt the user for the number of rows, n, and print the pattern accordingly. The resulting pattern aligns the numbers symmetrically.
Visual Presentation:
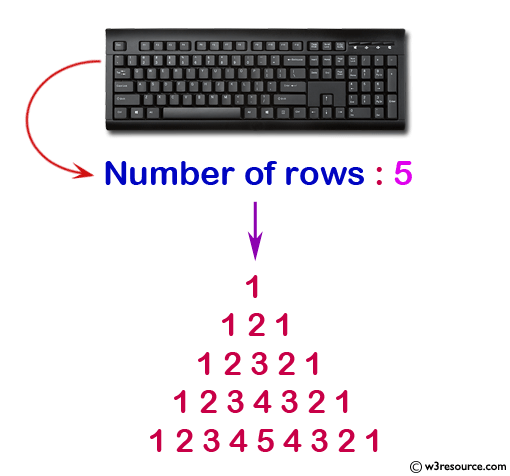
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, j, n; // Declare variables for loop counters and the number of rows.
printf("Input number of rows : "); // Prompt the user to input the number of rows.
scanf("%d", &n); // Read the input from the user.
for(i = 0; i <= n; i++) // Loop to generate each row of the pattern.
{
/* Print blank spaces */
for(j = 1; j <= n - i; j++) // Loop to print spaces before the numbers.
printf(" ");
/* Display numbers in ascending order up to the middle */
for(j = 1; j <= i; j++) // Loop to print numbers in ascending order.
printf("%d", j);
/* Display numbers in reverse order after the middle */
for(j = i - 1; j >= 1; j--) // Loop to print numbers in descending order.
printf("%d", j);
printf("\n"); // Move to the next line after printing a row.
}
}
Output:
Input number of rows : 5 1 121 12321 1234321 123454321
Flowchart:
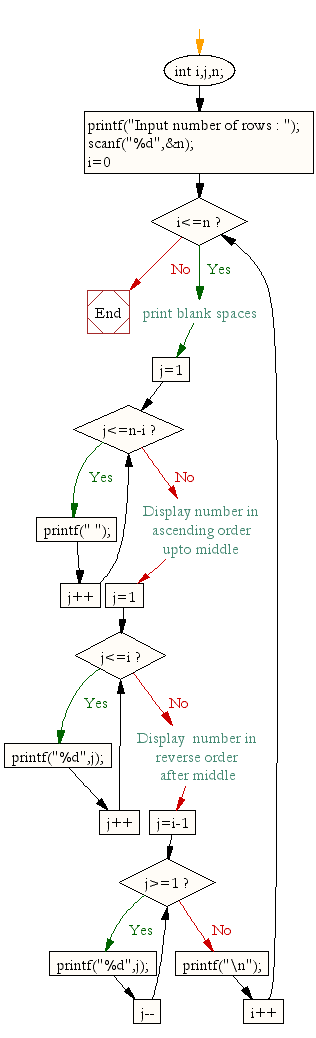
For more Practice: Solve these Related Problems:
- Write a C program to display a pattern for n rows where each row starts and ends with 1 and inner numbers increase.
- Write a C program to print a pattern with fixed 1’s on the border and incrementing numbers in the middle.
- Write a C program to generate a pyramid pattern where the first and last digits are 1 and the center values are computed recursively.
- Write a C program to display a pattern with 1’s at the boundaries and dynamic center values based on the row number.
C Programming Code Editor:
Previous: Write a program in C to display the first n terms of Fibonacci series.
Next: Write a program in C to display the number in reverse order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.