C Exercises: Convert Octal number to a Decimal number
51. Octal to Decimal Conversion (Without Array)
Write a C program to convert an octal number to a decimal without using an array.
This C program converts an octal number to a decimal without using an array. It reads an octal number from the user and checks if each digit is valid (less than 8). Then, it calculates the decimal equivalent of the octal number using a loop and displays the input octal number along with its decimal equivalent.
Visual Presentation:
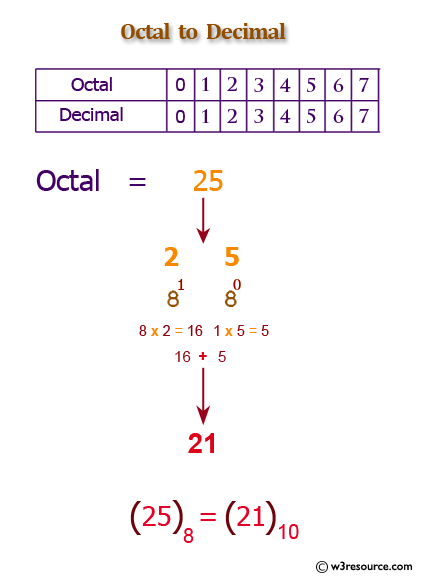
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int n1, n5, p = 1, k, ch = 1;
int dec = 0, i = 1, d;
printf("\n\nConvert Octal to Decimal:\n ");
printf("-------------------------\n");
printf("Input an octal number (using digits 0 - 7): ");
scanf("%d", &n1);
n5 = n1;
// Checking if any digit in the input number is greater than or equal to 8
for (; n1 > 0; n1 = n1 / 10) {
k = n1 % 10;
if (k >= 8) {
ch = 0;
break; // No need to continue checking if one invalid digit is found
}
}
// Switch-case to determine if the input is a valid octal number or not
switch (ch) {
case 0:
printf("\nThe number is not an octal number.\n\n");
break;
case 1:
n1 = n5;
// Calculating the decimal equivalent of the octal number
for (; n1 > 0; n1 = n1 / 10) {
d = n1 % 10;
dec += d * p;
p *= 8;
}
// Displaying the input octal number and its equivalent decimal number
printf("\nThe Octal Number: %d\nThe equivalent Decimal Number: %d\n\n", n5, dec);
break;
}
return 0;
}
Output:
Convert Octal to Decimal: ------------------------- Input an octal number (using digit 0 - 7) :745 The Octal Number : 745 The equivalent Decimal Number : 485
Explanation:
Here's a brief explanation.
- Header and Declaration:
- Includes the necessary header file (stdio.h).
- Declare variables 'n1' (input octal number), 'n5' (backup of the input), 'p' (power of 8 multiplier), 'k' (temporary variable for digit checking), 'ch' (flag for octal validity check), 'dec' (resulting decimal number), 'i' (loop variable), and 'd' (temporary variable for digit extraction).
- User Input:
- Asks the user to input an octal number (using digits 0 - 7).
- Reads the input and stores it in the variable 'n1'.
- Octal Validity Check:
- Uses a for loop to iterate through each digit of the input octal number ('n1').
- Checks if any digit is greater than or equal to 8, marking the number as invalid if true.
- Switch-Case for Octal Validity:
- Uses a switch statement based on the validity check ('ch').
- If invalid, prints a message indicating that the input is not an octal number.
- If valid, calculate the decimal equivalent.
- Decimal Equivalent Calculation:
- Reset 'n1' to the backup ('n5').
- Use a for loop to extract each digit of the octal number, multiplying it by the corresponding power of 8 ('p') and accumulating the result in the 'dec' variable.
- Result Display:
- Prints the input octal number and its equivalent decimal number.
- Return Statement:
- Returns 0 to indicate successful execution.
Flowchart:
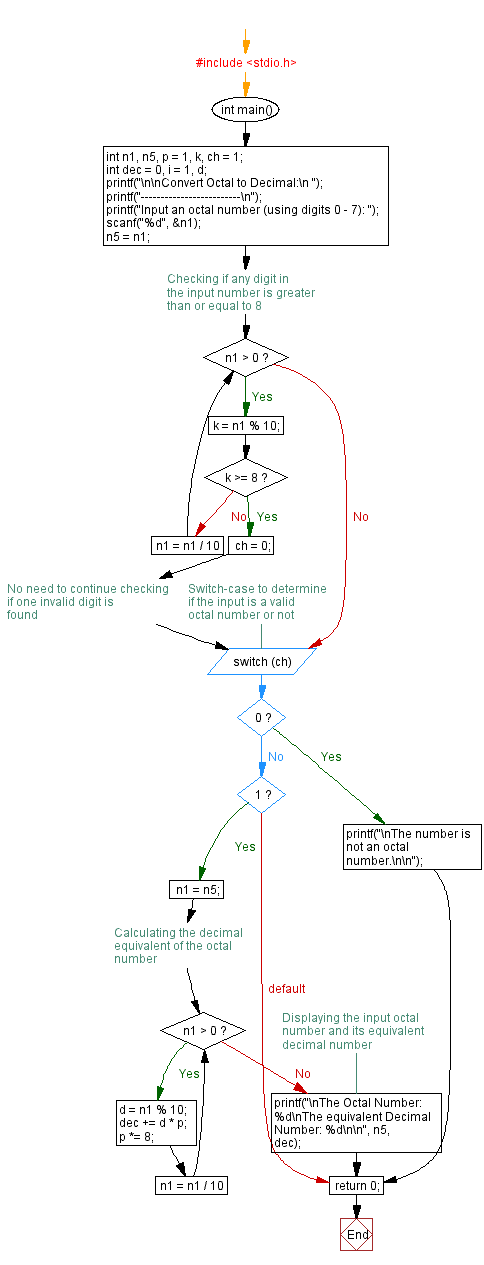
For more Practice: Solve these Related Problems:
- Write a C program to convert an octal number to decimal without using arrays by using arithmetic operations.
- Write a C program to convert an octal number to decimal without arrays and then display the sum of its digits.
- Write a C program to convert an octal number to decimal without using arrays and using recursion.
- Write a C program to convert an octal number to decimal and check if the resulting number is a palindrome.
C Programming Code Editor:
Previous: Write a program in C to convert a decimal number into octal without using an array.
Next: Write a program in c to find the Sum of GP series.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.