C Exercises: Find the Sum of GP series
C For Loop: Exercise-52 with Solution
Write a C program to find the sum of the G.P. series.
This C program calculates the sum of a geometric progression (G.P.) series. It takes the first term (g1), common ratio (cr), and the number of terms (ntrm) as input. Then, it iterates through each term, calculates the G.P. term using the formula, adds it to the sum, and prints each term. Finally, it prints the sum of the G.P. series.
Visual Presentation:
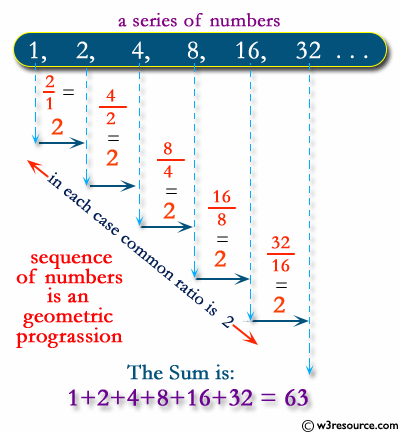
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
#include <math.h> // Include the math header file.
void main(){
float g1,cr,i,n,j;
float ntrm,gpn;
float sum=0;
// Get input for first term, number of terms and common ratio
printf("\\n\\n Find the Sum of GP series.:\\n ");
printf("-------------------------\\n");
printf("Input the first number of the G.P. series: ");
scanf("%f",&g1);
printf("Input the number or terms in the G.P. series: ");
scanf("%f",&ntrm);
printf("Input the common ratio of G.P. series: ");
scanf("%f",&cr);
// Generate G.P. series
printf("\\nThe numbers for the G.P. series:\\n ");
printf("%f ",g1);
sum=g1;
// Calculate and print G.P. terms
for(j=1;j<ntrm;j++){
gpn=g1*pow(cr,j);
sum=sum+gpn;
printf("%f ",gpn);
}
// Print sum of G.P. series
printf("\\nThe Sum of the G.P. series : %f\\n\\n",sum);
}
Output:
Input the first number of the G.P. series: 3 Input the number or terms in the G.P. series: 5 Input the common ratio of G.P. series: 2 The numbers for the G.P. series: 3.000000 6.000000 12.000000 24.000000 48.000000 The Sum of the G.P. series : 93.000000
Flowchart:
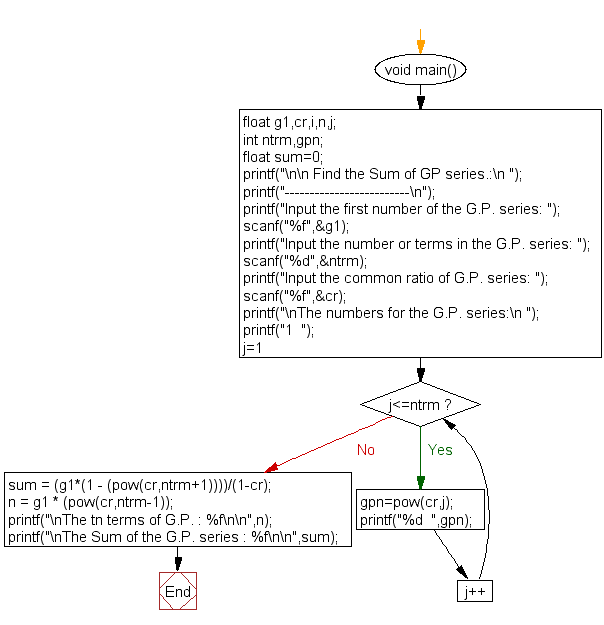
C Programming Code Editor:
Previous: Write a program in C to convert a Octal number to a Decimal number without using an array, function and while loop.
Next: Write a program in C to convert a binary number to octal.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics