C Exercises: Check whether a number can be express as sum of two prime numbers
56. Express Number as Sum of Two Primes
Write a program in C to check whether a number can be expressed as the sum of two prime.
This C program checks if a given number can be expressed as the sum of two prime numbers. It prompts the user to input a number, then iterates through all possible pairs of numbers up to half of the input number. For each pair, it checks if both numbers are prime. If both are prime, it prints the pair as the sum yielding the input number.
Visual Presentation:
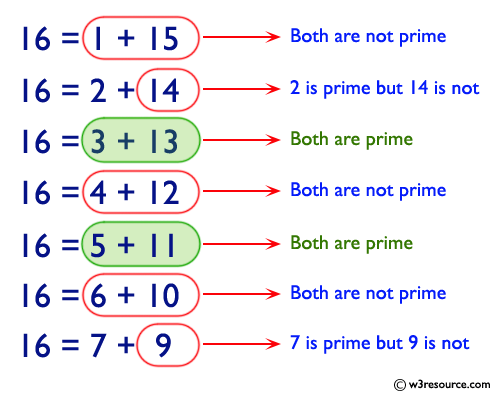
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
#include <stdlib.h>
#include <math.h> // Include the math header file.
int main()
{
// Variable declarations
int num, i, j, temp1, temp2, ctr=0;
// Prompting user for input
printf("input the number:\n");
// Getting user input
scanf("%d", &num);
// Loop to find pairs of numbers that add up to 'num'
for(i = 2; i<= num/2; i++){
temp1 = i;
temp2 = num - i;
// Checking if 'i' is a prime number
for(j = 2; j <= i/2; j++){
if(i % j == 0){
ctr++;
break;
}
}
// If 'i' is a prime number, check if 'num - i' is also prime
if(ctr == 0){
for(j = 2; j <= (num - i)/2; j++){
if((num - i) % j == 0){
ctr++;
break;
}
}
// If both 'i' and 'num - i' are prime, print the pair
if(ctr == 0)
printf("%d can be written as %d + %d.\n ", num, i, num - i);
}
// Resetting the counter
ctr = 0;
}
return 0;
}
Output:
input the number: 16 16 can be written as 3 + 13. 16 can be written as 5 + 11.
Flowchart:
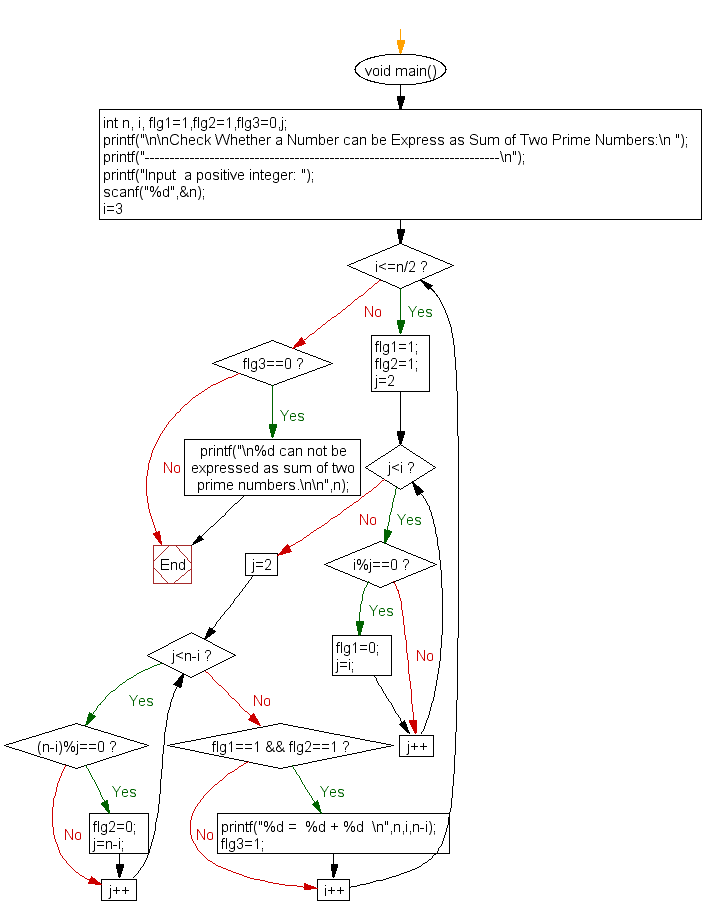
For more Practice: Solve these Related Problems:
- Write a C program to check if a given number can be expressed as the sum of two distinct prime numbers using nested loops.
- Write a C program to find all pairs of prime numbers that sum to a given number using the Sieve of Eratosthenes.
- Write a C program to determine if a number is the sum of two primes and output the pairs in ascending order.
- Write a C program to check if a number can be written as the sum of two primes and then display the difference between each prime pair.
C Programming Code Editor:
Previous: Write a program in C to convert a decimal number to hexadecimal.
Next: Write a program in C to print a string in reverse order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.