C Exercises: Print a string in reverse order
C For Loop: Exercise-57 with Solution
Write a C program to print a string in reverse order.
This C program takes a string as input, then reverses it by swapping characters from the leftmost position with the rightmost position iteratively until reaching the middle of the string. It prints the reversed string as the output.
Visual Presentation:
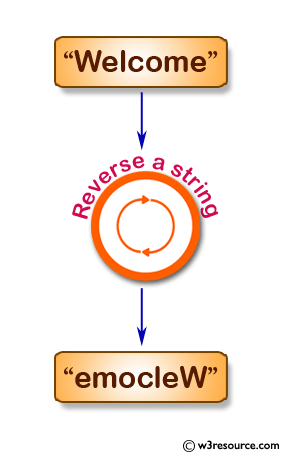
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
#include <string.h>
void main()
{
// Variable declarations
char str1[100], tmp;
int l, lind, rind, i;
// Prompting user for input
printf("\n\nPrint a string in reverse order:\n ");
printf("-------------------------------------\n");
// Getting user input
printf("Input a string to reverse : ");
scanf("%s", str1);
// Calculating the length of the string
l = strlen(str1);
// Initializing left and right indices for swapping
lind = 0;
rind = l - 1;
// Loop for swapping characters from left to right
for(i = lind; i< rind; i++)
{
tmp = str1[i];
str1[i] = str1[rind];
str1[rind] = tmp;
rind--;
}
// Printing the reversed string
printf("Reversed string is: %s\n\n", str1);
}
Output:
Print a string in reverse order: ------------------------------------- Input a string to reverse : Welcome Reversed string is: emocleW
Flowchart:
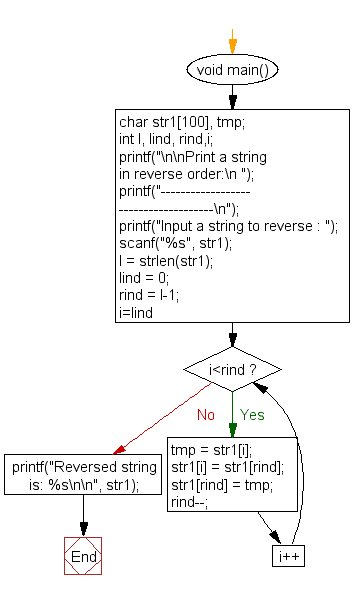
C Programming Code Editor:
Previous: Write a program in C to Check Whether a Number can be Express as Sum of Two Prime Numbers.
Next: Write a C program to find the length of a string without using the library function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics