C Exercises: Multiply two integers without using multiplication, division, bitwise operators, and loops
C Programming Mathematics: Exercise-20 with Solution
Write a C program to multiply two integers without using multiplication, division, bitwise operators, and loops.
Example 1:
Input: n1 = 50
Input: n2 = 12
Output: 600
Example 2:
Input: n1 = 0
Input: n2 = 12
Output: 0
Sample Solution:
C Code:
#include <stdio.h>
// Recursive function to multiply two integers 'a' and 'b'
int multiply_two_nums(int a, int b) {
/* 0 multiplied with anything gives 0 */
if (b == 0)
return 0; // Return 0 if 'b' is zero
if (b > 0)
return (a + multiply_two_nums(a, b - 1)); // Recursive call when 'b' is positive, using addition
if (b < 0)
return -multiply_two_nums(a, -b); // Recursive call when 'b' is negative, using recursion and negation
return -1; // Return -1 for invalid inputs
}
// Main function to test the multiply_two_nums function with different values
int main(void) {
int n1 = 50;
int n2 = 12;
printf("\n %d x %d = %d", n1, n2, multiply_two_nums(n1, n2)); // Print the result of multiplication
n1 = 0;
n2 = 12;
printf("\n %d x %d = %d", n1, n2, multiply_two_nums(n1, n2)); // Print the result of multiplication
return 0;
}
Sample Output:
50 x 12 = 600 0 x 12 = 0
Flowchart:
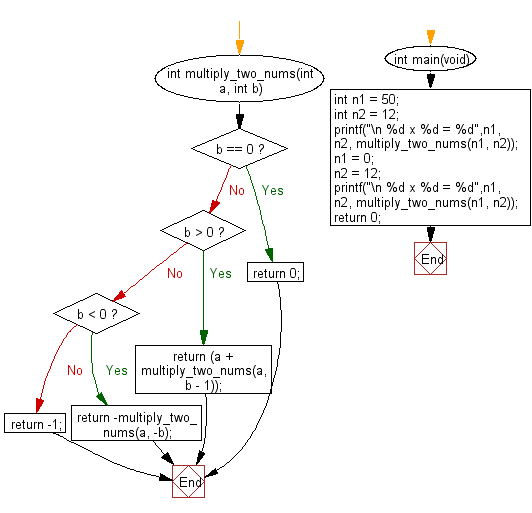
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to find the square root of a number using Babylonian method.
Next: Write a C program to calculate and print average (or mean) of the stream of given numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/math/c-math-exercise-20.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics