C Exercises: Count the numbers without digit 7, from 1 to a given number
22. Count Numbers Without Digit 7 Variants
Write a C program to count the numbers without the digit 7, from 1 to a given number.
Example 1:
Input: n = 10
Output: 9
Example 2:
Input: n = 687
Output: 555
Visual Presentation:
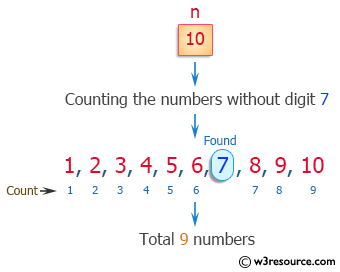
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function to count the numbers without digit 7
int count_nums_not_7(int num) {
if (num < 7)
return num; // If the number is less than 7, return the number itself
if (num >= 7 && num < 10)
return num - 1; // If the number is 7 or 8 or 9, return num - 1
int r = 1;
while (num / r > 9)
r = r * 10; // Find the divisor 'r' to split the number
int m = num / r; // Extract the most significant digit of the number
if (m != 7)
return count_nums_not_7(m) * count_nums_not_7(r - 1) + count_nums_not_7(m) + count_nums_not_7(num % r);
else
return count_nums_not_7(m * r - 1);
}
// Main function
int main(void) {
int n = 10;
if (n > 0)
printf("Count the numbers without digit 7, from 1 to %d : %d", n, count_nums_not_7(n));
n = 687;
if (n > 0)
printf("\nCount the numbers without digit 7, from 1 to %d : %d", n, count_nums_not_7(n));
return 0; // End of the main function
}
Sample Output:
Count the numbers without digit 7, from 1 to 10 : 9 Count the numbers without digit 7, from 1 to 687 : 555
Flowchart:
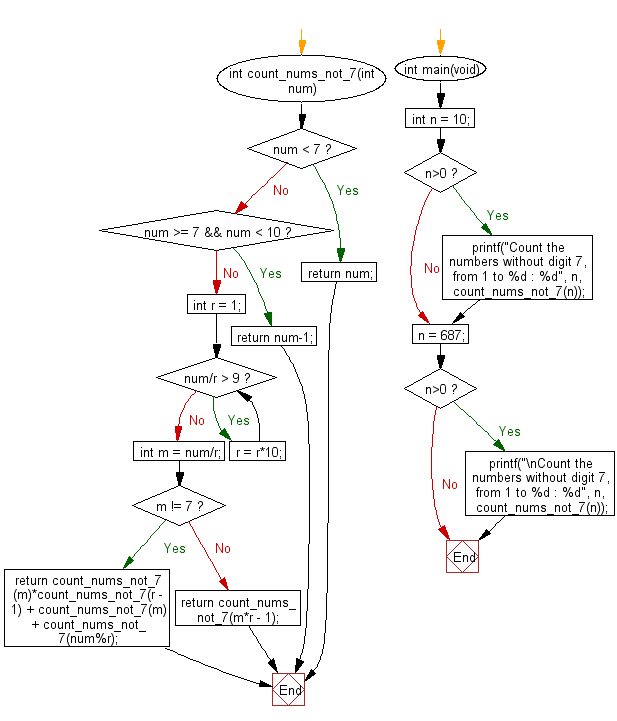
For more Practice: Solve these Related Problems:
- Write a C program to count all numbers from 1 to n that do not contain the digit 7 by checking each digit.
- Write a C program to optimize the count of numbers missing digit 7 using mathematical pattern analysis.
- Write a C program to iterate through numbers and skip those containing 7 using string conversion.
- Write a C program to count numbers without the digit 7 recursively by dividing the range into segments.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to calculate and print average (or mean) of the stream of given numbers.
Next: Write a C program to find next smallest palindrome of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.