C Exercises: Find next smallest palindrome of a given number
C Programming Mathematics: Exercise-23 with Solution
Write a C program to find next smallest palindrome of a given number.
From Wikipedia,
A palindrome is a word, number, phrase, or other sequence of characters which reads the same backward as forward, such as madam, racecar. There are also numeric palindromes, including date/time stamps using short digits 11/11/11 11:11 and long digits 02/02/2020. Sentence-length palindromes may be written when allowances are made for adjustments to capital letters, punctuation, and word dividers, such as "A man, a plan, a canal, Panama!".
Example:
Input: n = 121
Output: Next smallest palindrome of 121 is 131
Visual Presentation:
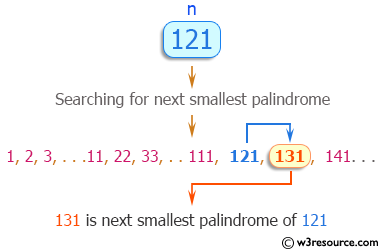
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function to generate the next smallest palindrome after 'n'
int nextPalindromeGenerate(int n)
{
int ans=1, digit, rev_num=0, num; // Declare variables ans, digit, rev_num, and num
if(n<10) // Check if n is less than 10
{
ans=0; // Set ans to 0
return n+1; // Return n+1 as the next smallest palindrome
}
num=n; // Store the original value of n
while(ans!=0) // While loop until ans is not 0
{
rev_num=0; // Initialize rev_num to 0
digit=0; // Initialize digit to 0
n=++num; // Increment num and assign to n
while(n>0) // While loop to reverse the number and store it in rev_num
{
digit=n%10; // Get the last digit of n
rev_num=rev_num*10+digit; // Append digit to rev_num
n=n/10; // Remove the last digit from n
}
if(rev_num==num) // Check if the reversed number is equal to the original number
{
ans=0; // Set ans to 0
return num; // Return the palindrome number
}
else
ans=1; // Set ans to 1 to continue the loop
}
return num; // Return the next palindrome number
}
int main(void)
{
int n = 121; // Declare and initialize n to 121
if (n>0); // Check if n is positive (semicolon here may cause unexpected behavior, it's better to remove it)
{
printf("Next smallest palindrome of %d is %d", n, nextPalindromeGenerate(n)); // Print the next smallest palindrome of n
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Next smallest palindrome of 121 is 131
Flowchart:
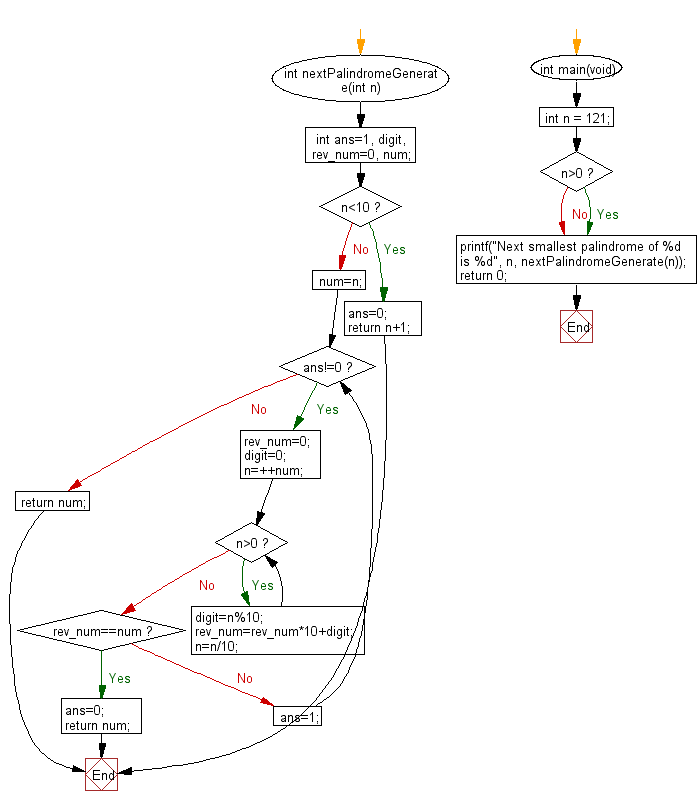
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to count the numbers without digit 7, from 1 to a given number.
Next: Write a C program to calculate e raise to the power x using sum of first n terms of Taylor Series.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/math/c-math-exercise-23.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics