C Exercises: Display the first 10 lucus numbers
11. Lucas Numbers Variants
Write a program in C to display the first 10 lucus numbers.
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
int main()
{
printf("\n\n Find the first 10 Lucas numbers: \n"); // Print message to find Lucas numbers
printf(" -------------------------------------\n"); // Print separator
printf(" The first 10 Lucas numbers are: \n"); // Print message
int n = 10; // Number of Lucas numbers to generate
int n1 = 2, n2 = 1, n3; // Initialize the first two Lucas numbers
if (n > 1) // Check if the number of Lucas numbers is greater than 1
{
printf("%d %d ", n1, n2); // Print the first two Lucas numbers
for (int i = 2; i < n; ++i) // Loop to generate remaining Lucas numbers
{
n3 = n2; // Store the value of n2 in n3
n2 += n1; // Calculate the next Lucas number by adding the previous two
n1 = n3; // Update n1 to the previous n2
printf("%d ", n2); // Print the current Lucas number
}
printf("\n"); // Print newline after printing all Lucas numbers
}
else if (n == 1) // Check if only one Lucas number is to be generated
{
printf("%d ", n2); // Print the first Lucas number
printf("\n"); // Print newline after printing the Lucas number
}
else // If input is less than 1
{
printf("Input a positive number.\n"); // Print message to input a positive number
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
The first 10 Lucus numbers are: 2 1 3 4 7 11 18 29 47 76
Flowchart:
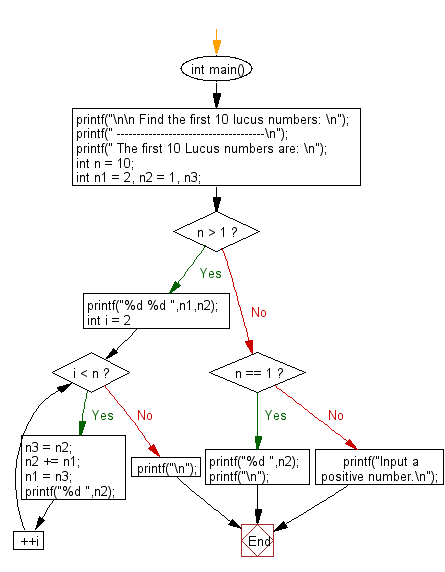
For more Practice: Solve these Related Problems:
- Write a C program to generate Lucas numbers using an iterative approach.
- Write a C program to compute Lucas numbers recursively with memoization for speed.
- Write a C program to generate Lucas numbers and compare them with Fibonacci numbers.
- Write a C program to display Lucas numbers in reverse order.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to generate and show the first 15 narcissistic decimal numbers.
Next: Write a program in C to display the first 10 catlan numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.