C Exercises: Generate and show the first 15 narcissistic decimal numbers
10. Narcissistic Numbers Variants
Write a program in C to generate and show the first 15 narcissistic decimal numbers.
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
int main()
{
printf("\n\n Find the first 15 narcissistic decimal numbers: \n");
printf(" -----------------------------------------------------\n");
printf(" The first 15 narcissistic decimal numbers are: \n");
int i, ctr, j, orn, n, m, sum;
orn = 1; // Initialize the original number to check
for (i = 1; i <= 15;) // Loop to find the first 15 narcissistic decimal numbers
{
ctr = 0; // Initialize digit counter for each number
sum = 0; // Initialize sum of digits raised to power 'ctr'
n = orn; // Assign original number to 'n'
// Count the number of digits in the number 'n'
while (n > 0)
{
n = n / 10;
ctr++;
}
n = orn; // Reset 'n' to the original number
// Calculate the sum of digits raised to power 'ctr'
while (n > 0)
{
m = n % 10; // Extract individual digits
sum = sum + pow(m, ctr); // Add the digit raised to the power 'ctr' to 'sum'
n = n / 10; // Move to the next digit
}
// Check if the sum of digits raised to power 'ctr' equals the original number
if (sum == orn)
{
printf("%d ", orn); // Print the narcissistic number
i++; // Increment the count of narcissistic numbers found
}
orn++; // Move to the next number to check
}
printf("\n");
return 0;
}
Sample Output:
The first 15 narcissistic decimal numbers are: 1 2 3 4 5 6 7 8 9 153 370 371 407 1634 8208
Visual Presentation:
Flowchart:
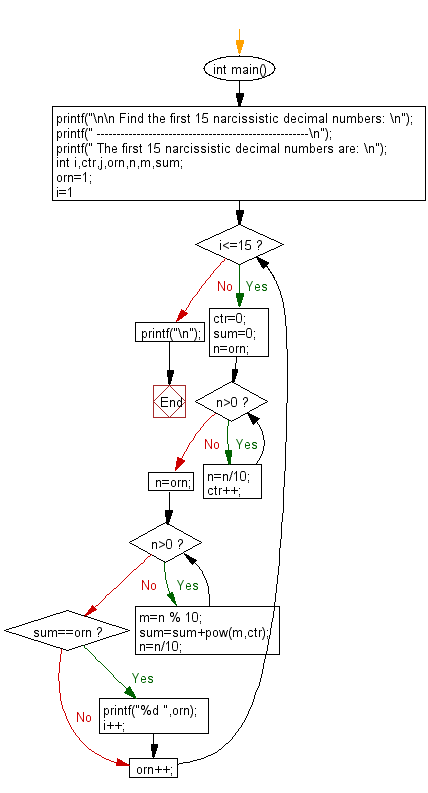
For more Practice: Solve these Related Problems:
- Write a C program to generate narcissistic numbers for numbers with varying digit lengths.
- Write a C program to check if a number is narcissistic by summing the powers of its digits.
- Write a C program to list the first n narcissistic numbers based on user input.
- Write a C program to verify narcissistic numbers using recursive digit extraction.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to display and count the number of Lychrel numbers within a specific range(from 1 to a specific upper limit).
Next: Write a program in C to display the first 10 lucus numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.