C Exercises: Display and count the number of Lychrel numbers within a specific range.
9. Lychrel Numbers in a Range Variants
Write a program in C to display and count the number of Lychrel numbers within a specific range (from 1 to a specific upper limit).
Test Data
Input the upper limit: 1000
Sample Solution:
C Code:
#include <stdio.h> // Include standard input-output library
#include <stdbool.h> // Include library for boolean type
#include <stdlib.h> // Include standard library
// Function to check if a number is a palindrome
// Function prototypes
bool palindrome(unsigned long long int i); // Function to check if a number is a palindrome
unsigned long long int reverse(unsigned long long int i); // Function to reverse a number
bool lychrel(unsigned long long int i); // Function to check if a number is a Lychrel number
int main(void) {
unsigned long long int i = 0; // Declare and initialize variable for input number
int count = 0, ulmt; // Declare variables for count and upper limit
printf("\n\n Display and count number of Lychrel numbers within a specific range: \n"); // Print message
printf(" -------------------------------------------------------------------------\n"); // Print separator
printf(" Input the upper limit: "); // Prompt user for input
scanf("%d", &ulmt); // Read upper limit from user
printf("\n The Lychrel numbers are: \n"); // Print message
for (i = 1; i < ulmt; i++) { // Loop through numbers up to upper limit
if (lychrel(i)) { // Check if the number is a Lychrel number
printf(" %llu ", i); // Print the Lychrel number
count++; // Increment count
}
}
printf("\n The number of Lychrel numbers are: %d\n\n", count); // Print the count of Lychrel numbers
return 0; // Return 0 to indicate successful execution
}
// Function to check if a number is a Lychrel number
bool lychrel(unsigned long long int i) {
int j; // Declare variable for iteration counter
bool lychrel = true; // Initialize variable to store Lychrel property
i = i + reverse(i); // Add the input number to its reverse
for (j = 1; j <= 30; j++) { // Loop up to 30 iterations
if (palindrome(i)) { // Check if the result is a palindrome
lychrel = false; // If palindrome found, set lychrel to false
break; // Exit the loop
}
i = i + reverse(i); // Add the number to its reverse for the next iteration
}
return lychrel; // Return the Lychrel property
}
// Function to reverse a number
unsigned long long int reverse(unsigned long long int i) {
unsigned long long int ret = 0; // Declare and initialize variable to store reversed number
while (i != 0) { // While loop to reverse the number
ret *= 10; // Multiply ret by 10
ret += i % 10; // Add the last digit of i to ret
i /= 10; // Remove the last digit of i
}
return ret; // Return the reversed number
}
// Function to check if a number is a palindrome
bool palindrome(unsigned long long int i) {
return (i == reverse(i)); // Return true if i is equal to its reverse, otherwise return false
}
Sample Output:
Input the upper limit: 1000 The Lychrel numbers are: 196 295 394 493 592 689 691 788 790 879 887 978 986 The number of Lychrel numbers are: 13
Visual Presentation:
Flowchart:
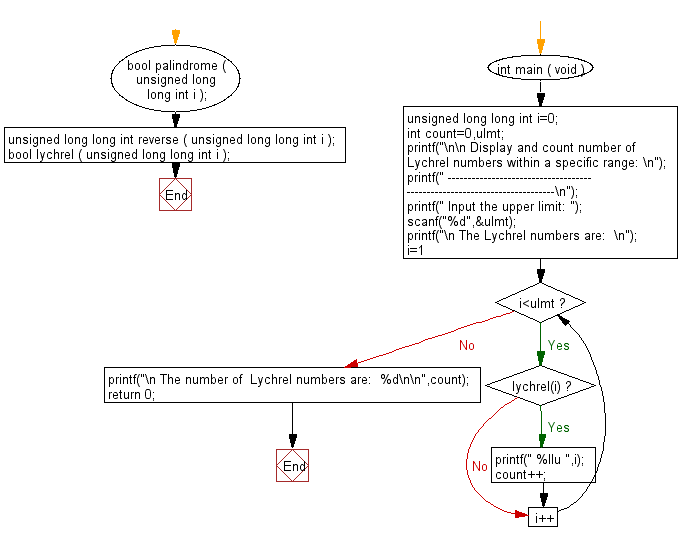
For more Practice: Solve these Related Problems:
- Write a C program to list all suspected Lychrel numbers between two bounds.
- Write a C program to count Lychrel numbers within a user-defined interval and output the total.
- Write a C program to check each candidate’s reverse-add sequence and print the iteration steps.
- Write a C program to filter and display Lychrel numbers from an array using optimized loops.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check whether a number is Lychrel number or not.
Next: Write a program in C to generate and show the first 15 narcissistic decimal numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.