C Exercises: Check if a given number is circular prime or not
27. Circular Prime Check Variants
Write a program in C to check if a given number is circular prime or not.
Test DataInput a Number: 1193
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
# include <stdbool.h>
# include <math.h>
int flg; // Global variable to store the flag indicating if the number is a circular prime or not
// Function to check if a number is prime
void chkPrime(long int n)
{
long int i = n - 1; // Initialize 'i' to check divisibility of 'n'
while (i >= 2) // Loop to check for divisibility of 'n' by numbers less than 'n'
{
if (n % i == 0) // If 'n' is divisible by 'i', set flag to 1 (not a prime number)
{
flg = 1;
}
i--; // Decrement 'i' to check the next number
}
}
// Function to find all combinations of digits in the number and check for prime
void AllCombination(long int a)
{
long int b1, c1, d1, e1, i, j, k, s1, z1, v1, x[8], y[8], m;
b1 = a;
i = 0;
// Extracting digits of the number and storing in an array 'y'
while (b1 > 0)
{
y[i] = b1 % 10;
b1 = b1 / 10;
i++;
}
c1 = 0;
// Reversing the order of digits and storing in array 'x'
for (j = i - 1; j >= 0; j--)
{
x[c1] = y[j];
c1++;
}
m = i;
// Generating all possible combinations of digits
while (m > 0)
{
c1 = m - 1;
d1 = i - 1;
e1 = 0;
s1 = 0;
while (e1 < i)
{
z1 = pow(10, d1);
v1 = z1 * x[c1 % i];
c1++;
d1--;
e1++;
s1 = s1 + v1;
}
m--;
// Checking if the generated number is prime
chkPrime(s1);
}
}
// Main function
int main()
{
long int num1; // Variable to store the input number
// Printing information about the program and asking for user input
printf("\n\n Check whether a given number is a circular prime or not: \n");
printf(" -----------------------------------------------------------\n");
printf(" Input a Number: ");
scanf("%li", &num1);
flg = 0; // Resetting flag to 0
AllCombination(num1); // Calling function to check circular prime
if (flg == 0)
{
printf(" The given number is a circular prime Number.\n"); // Printing if the number is a circular prime
}
else
{
printf(" The given number is not a circular prime Number.\n"); // Printing if the number is not a circular prime
}
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Input a Number: 1193 The given number is a circular prime Number.
Visual Presentation:
Flowchart:
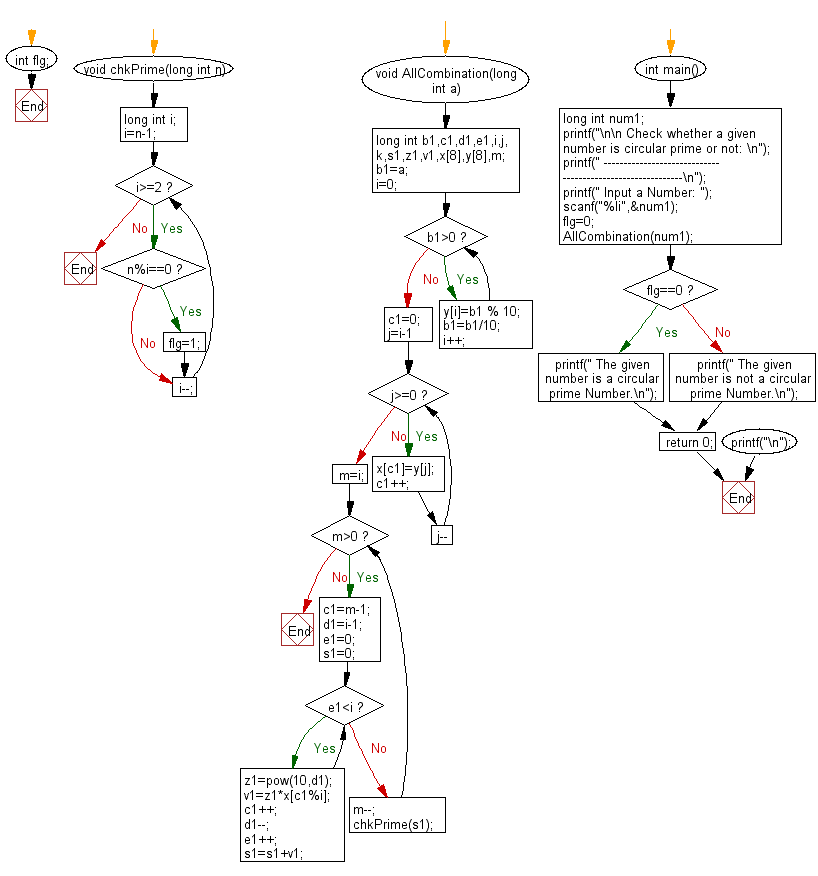
For more Practice: Solve these Related Problems:
- Write a C program to check if a number is a circular prime by rotating its digits and testing each rotation.
- Write a C program to verify circular primes using an optimized prime-checking function.
- Write a C program to list circular primes along with all their rotational variants.
- Write a C program to test circular prime properties in a given range with early exit on failure.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to count the amicable pairs in an array.
Next: Write a program in C to find circular prime numbers upto a specific limit.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.