C Exercises: Count the amicable pairs in an array
26. Count Amicable Pairs in Array Variants
Write a program in C to count the amicable pairs in an array.
Test DataInput the number of elements to be stored in the array: 4
element - 0: 220
element - 1: 274
element - 2: 1184
element - 3: 1210
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
# include <stdbool.h>
# include <math.h>
// Function to find the sum of proper divisors of a number 'n'
int ProDivSum(int n)
{
int sum = 1;
for (int i = 2; i <= sqrt(n); i++) // Loop to find divisors of 'n'
{
if (n % i == 0)
{
sum += i; // Adding divisor 'i' to the sum
if (n / i != i)
sum += n / i; // Adding the corresponding divisor to the sum
}
}
return sum; // Returning the sum of divisors
}
// Function to check if two numbers 'a' and 'b' are Amicable pairs
bool chkAmicable(int a, int b)
{
return (ProDivSum(a) == b && ProDivSum(b) == a); // Checking if the sums of divisors of 'a' and 'b' match each other
}
// Function to count the number of Amicable pairs in an array
int ChkPairs(int arr[], int num1)
{
int ctr = 0;
for (int i = 0; i < num1; i++) // Loop through the elements of the array
{
for (int j = i + 1; j < num1; j++) // Loop through subsequent elements in the array
{
if (chkAmicable(arr[i], arr[j])) // Checking if the pair (arr[i], arr[j]) is an Amicable pair
ctr++; // Incrementing the counter if an Amicable pair is found
}
}
return ctr; // Returning the count of Amicable pairs
}
// Main function
int main()
{
int nn; // Variable to store the number of elements in the array
// Printing information about the program and asking for user input
printf("\n\n Count the Amicable pairs in a specific array: \n");
printf(" Sample pairs : (220, 284)(1184,1210) (2620,2924) (5020,5564) (6232,6368)... \n");
printf(" ------------------------------------------------------------------------------\n");
printf("\n Input the number of elements to be stored in the array: ");
scanf("%d", &nn);
int arr1[nn]; // Array to store elements entered by the user
// Taking input of elements to be stored in the array
for (int i = 0; i < nn; i++)
{
printf(" element - %d: ", i);
scanf("%d", &arr1[i]);
}
int n1 = sizeof(arr1) / sizeof(arr1[0]); // Calculating the number of elements in the array
// Displaying the number of Amicable pairs present in the array
printf(" Number of Amicable pairs presents in the array: %d\n", ChkPairs(arr1, n1));
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Input the number of elements to be stored in the array: 4 element - 0: 220 element - 1: 274 element - 2: 1184 element - 3: 1210 Number of Amicable pairs presents in the array: 1
Flowchart:
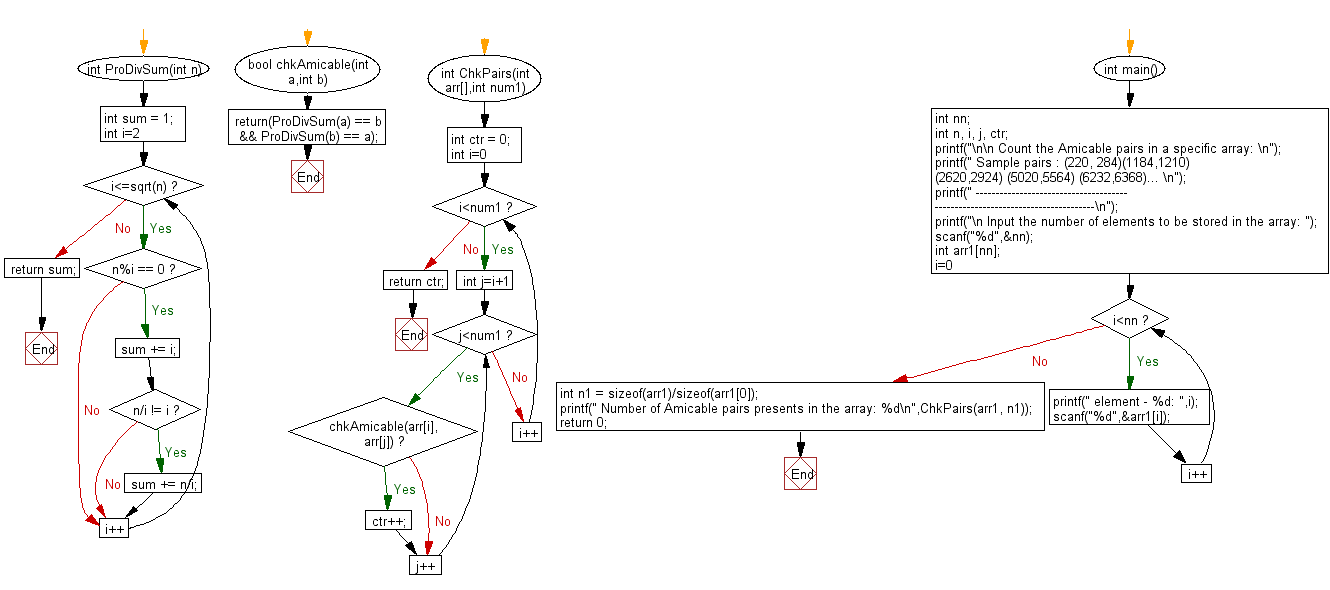
For more Practice: Solve these Related Problems:
- Write a C program to count amicable pairs in an array and list each pair with divisor details.
- Write a C program to optimize the search for amicable pairs using precomputed divisor sum arrays.
- Write a C program to implement amicable pair detection in an unsorted array with efficient lookups.
- Write a C program to count and display the index positions of amicable pairs found in an array.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check two numbers are Amicable numbers or not.
Next: Write a program in C to check if a given number is circular prime or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.