C Exercises: Total of two indices values equal a target
1. Two-Sum Indices Variants
Write a C program to get the indices of two numbers in a given array of integers. This will enable you to get the sum of two numbers equal to a specific target.
C Code:
#include <stdio.h>
#include <stdlib.h>
struct object {
int val;
int index;
};
static int compare(const void *a, const void *b)
{
return ((struct object *) a)->val - ((struct object *) b)->val;
}
static int * two_sum(int *nums, int nums_size, int target)
{
int i, j;
struct object *objes = malloc(nums_size * sizeof(*objes));
for (i = 0; i < nums_size; i++) {
objes[i].val = nums[i];
objes[i].index = i;
}
qsort(objes, nums_size, sizeof(*objes), compare);
int *results = malloc(2 * sizeof(int));
i = 0;
j = nums_size - 1;
while (i < j) {
int diff = target - objes[i].val;
if (diff > objes[j].val) {
while (++i < j && objes[i].val == objes[i - 1].val) {}
} else if (diff < objes[j].val) {
while (--j > i && objes[j].val == objes[j + 1].val) {}
} else {
results[0] = objes[i].index;
results[1] = objes[j].index;
return results;
}
}
return NULL;
}
int main(void)
{
int nums[] = { 4, 2, 1, 5 };
int ctr = sizeof(nums) / sizeof(*nums);
int target = 7;
int i;
printf("Original Array: ");
for(i=0; i<ctr; i++)
{
printf("%d ", nums[i]);
}
printf("\nTarget Value: %d", target);
int *indexes = two_sum(nums, ctr, target);
if (indexes != NULL) {
printf("\nIndices of the two numbers whose sum equal to target value: %d", target);
printf("\n%d %d\n", indexes[0], indexes[1]);
}
else
{
printf("Not found or matched\n");
}
return 0;
}
Sample Output:
Original Array: 4 2 1 5 Target Value: 7 Indices of the two numbers whose sum equal to target value: 7 1 3
Pictorial Presentation:
Flowchart:
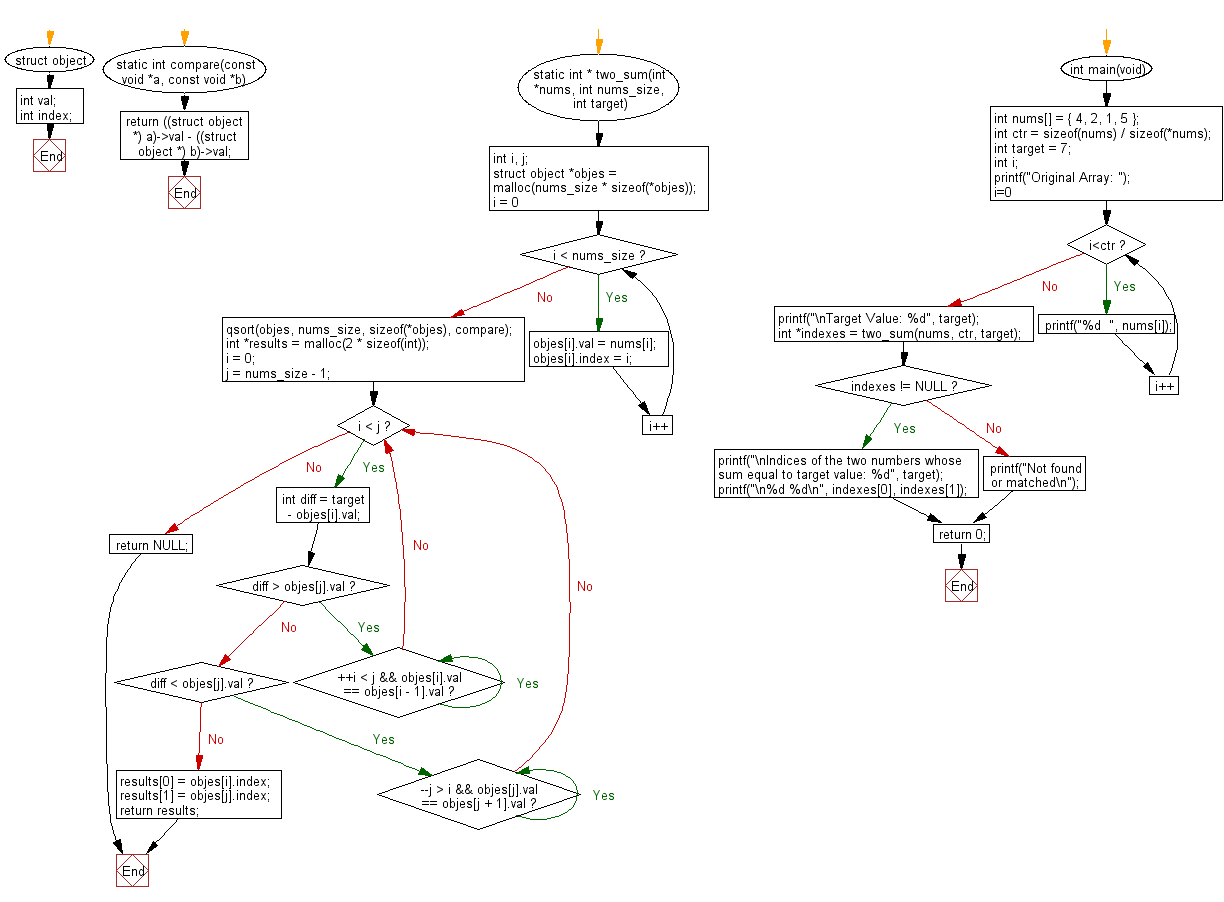
For more Practice: Solve these Related Problems:
- Write a C program to find indices of two numbers in an unsorted array that sum to a given target using a hash table.
- Write a C program to locate indices of two numbers in a sorted array whose sum equals the target using binary search for complements.
- Write a C program to identify indices of two numbers in a circular array that sum to a specified target.
- Write a C program to find indices of two numbers that sum to a target, ensuring the solution returns the smallest possible index pair.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: C programming Exercises Home
Next C Programming Exercise: No repeating characters in a substring.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.