C Exercises: No repeating characters in a substring
C Programming Challenges: Exercise-2 with Solution
Write a C program to find the length of the longest substring of a given string without repeating characters.
Note:
1) Given string consists of English letters, digits, symbols and spaces.
2) 0 <= Given string length <= 5 * 104
Difficulty: Medium.
Company: Amazon, Google, Bloomberg, Microsoft, Adobe, Apple, Oracle, Facebook and more.
Input String : xyzxyzyy
The longest Substring Length : 3
The longest substring : xyz
Input String : pickoutthelongestsubstring
The longest Substring Length : 8
The longest substring : ubstring
Input String : ppppppppppppp
The longest substring : p
The longest Substring Length : 1
Input String : Microsoft
The longest substring : Micros
The longest Substring Length : 6
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
static int length_of_longest_substring(char *str1)
{
int offset[128];
int substr_max_len = 0;
int len = 0;
int index = 0;
memset(offset, 0xff, sizeof(offset));
while (*str1 != '\0') {
if (offset[*str1] == -1) {
len++;
}
else {
if (index - offset[*str1] > len)
{
len++;
}
else
{
len = index - offset[*str1];
}
}
if (len > substr_max_len)
{
substr_max_len = len;
}
offset[*str1++] = index++;
}
return substr_max_len;
}
int main(void)
{
char *str1 = "xyzxyzyy";
printf("\nOriginal String: %s", str1);
printf("\nLength of the longest substring without repeating characters: %d\n", length_of_longest_substring(str1));
return 0;
}
Sample Output:
Original String: xyzxyzyy Length of the longest substring without repeating characters: 3
Pictorial Presentation:
Flowchart:
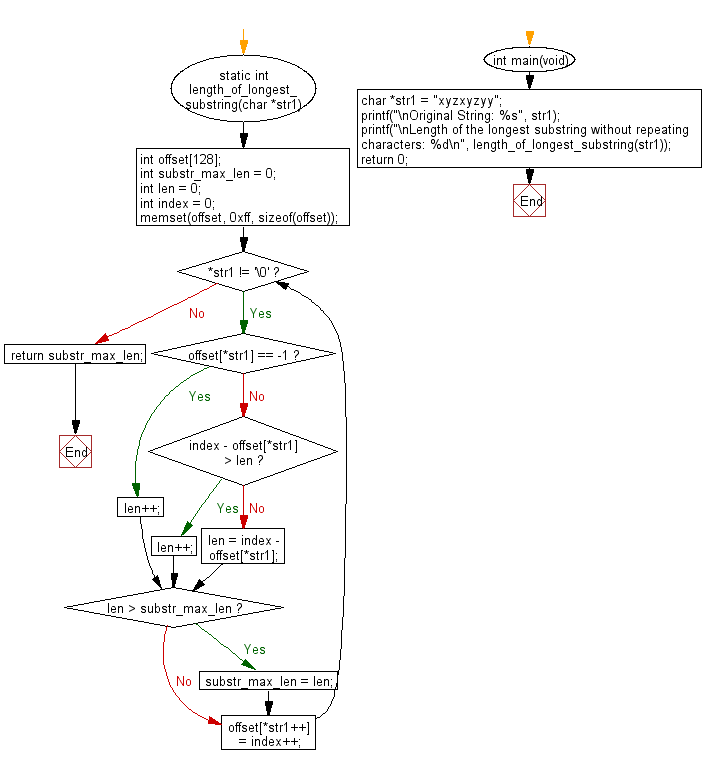
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Total of two indices values equal a target.
Next C Programming Exercise: Median of two non-empty sorted arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/practice/c-programming-practice-exercises-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics