C Exercises: Remove duplicates from an array of integers
12. Remove Duplicates from Sorted Array Variants
Write a C program to remove duplicates from a given array of integers.
C Code:
#include <stdio.h>
#include <stdlib.h>
static int remove_duplicates(int* nums, int arr_size)
{
if (arr_size <= 1) {
return arr_size;
}
int i = 0, j, count = 1;
while (i < arr_size) {
for (j = i + 1; j < arr_size && nums[i] == nums[j]; j++) {}
if (j < arr_size) {
nums[count++] = nums[j];
}
i = j;
}
return count;
}
int main(void)
{
int nums[] = {1,1,2,3,4,4,5,6,6,6};
int size = sizeof(nums)/sizeof(nums[0]);
printf("Original array:\n");
int i;
for (i = 0; i < size; i++) {
printf("%d ", nums[i]);
}
int count = remove_duplicates(nums, size);
printf("\nAfter removing duplicates from the above sorted array:\n");
for (i = 0; i < count; i++) {
printf("%d ", nums[i]);
}
printf("\n");
return 0;
}
Sample Output:
Original array: 1 1 2 3 4 4 5 6 6 6 After removing duplicates from the above sorted array: 1 2 3 4 5 6
Pictorial Presentation:
Flowchart:
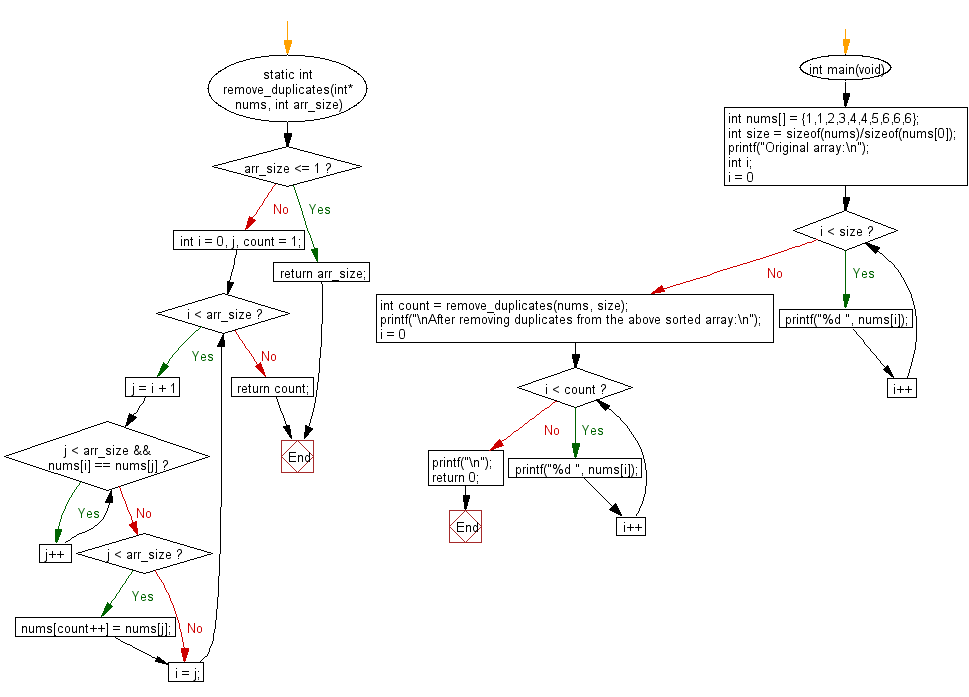
For more Practice: Solve these Related Problems:
- Write a C program to remove duplicates from a sorted array in-place and return the new length.
- Write a C program to remove duplicates from a sorted linked list and display the unique list.
- Write a C program to output unique elements from a sorted array after removing duplicates.
- Write a C program to implement duplicate removal from a sorted array using a two-pointer approach.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Combinations of parentheses from n pairs.
Next C Programming Exercise: Array length, remove instances of a value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.