C Exercises: Sum of all the multiples of 3 or 7 below 100
17. Sum of Multiples of 3 or 7 Below 100 Variants
The natural numbers below 10 that are multiples of 3 or 7 are 3, 7, 6 and 9. The sum of these multiples is 25.
Write a C programming to find the sum of all the multiples of 3 or 7 below 100.
C Code:
#include <stdio.h>
int main(void)
{
int sum3 = 0, sum7 = 0, sum21 = 0;
int i;
for (i = 0; i < 100; i++) {
if (i % 3 == 0) {
sum3 += i;
}
if (i % 7 == 0) {
sum7 += i;
}
if (i % 21 == 0) {
sum21 += i;
}
}
printf("%d\n", sum3 + sum7 - sum21);
return 0;
}
Sample Output:
2208
Flowchart:
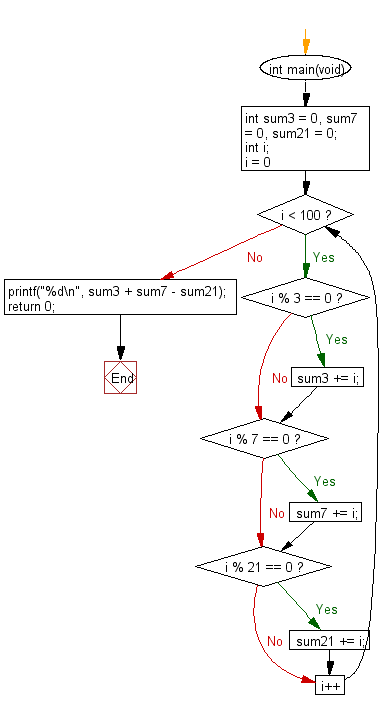
For more Practice: Solve these Related Problems:
- Write a C program to list all multiples of 3 or 7 below 100 and then compute their sum.
- Write a C program to compute the sum of multiples of 3 or 7 below a user-specified number using recursion.
- Write a C program to calculate the sum of multiples of 3 or 7 below 100 using a mathematical formula.
- Write a C program to compute and display the sum and count of multiples of 3 or 7 below 100.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Length, longest valid parentheses substring.
Next C Programming Exercise: Sum even-valued in a Fibonacci sequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.