C Exercises: Largest palindrome from two 3-digit numbers
20. Largest Palindrome Product Variants
A palindrome is a word, number, phrase, or other sequence of characters which reads the same backward as forward, such as taco cat or madam or racecar or the number 10801. The largest palindrome made from the product of two 2-digit numbers is 9009 = 91 × 99.
Write a C programming to find the largest palindrome made from the product of two 3-digit numbers.
C Code:
#include <stdio.h>
static int test_palindromic(unsigned int n);
int main(void)
{
unsigned int i, j, max = 0;
for (i = 100; i <= 999; i++) {
for (j = 100; j <= 999; j++) {
unsigned int p = i*j;
if (test_palindromic(p) && p > max) {
max = p;
}
}
}
printf("%u\n", max);
return 0;
}
int test_palindromic(unsigned int n)
{
unsigned int reversed_num = 0, t = n;
while (t) {
reversed_num = 10*reversed_num + (t % 10);
t /= 10;
}
return reversed_num == n;
}
Sample Output:
906609
Flowchart:
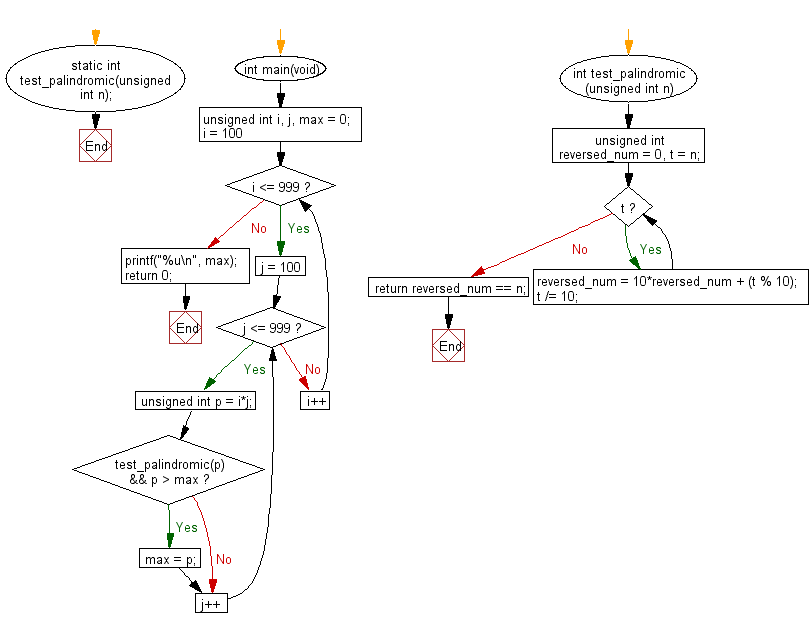
For more Practice: Solve these Related Problems:
- Write a C program to find the largest palindrome from the product of two 3-digit numbers using brute-force search.
- Write a C program to optimize the search for the largest palindrome by iterating downward.
- Write a C program to return both the largest palindrome and the pair of 3-digit factors that form it.
- Write a C program to verify the palindrome property of a product and then output the corresponding factor pair.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Find largest prime factor of 438927456.
Next C Programming Exercise: Smallest positive number divisible by 1-20.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.