C Exercises: Find the product xyz
25. First Missing Positive Integer Variants
A Pythagorean triplet is a set of three natural numbers, x < y < z, for which,
x2 + y2 = z2
For example, 32 + 42 = 9 + 16 = 25 = 52.
There exists exactly one Pythagorean triplet for which x +y + z = 1000.
Write a C program to find the product of xyz.
C Code:
#include <stdio.h>
#include <stdio.h>
int main(void)
{
int x, y;
for (x = 1; x <= 333; x++) {
for (y = x; y <= 666; y++) {
int z = (1000 - x - y);
if (x*x + y*y == z*z) {
printf("Numbers are: %d, %d, %d ", x, y, z);
printf("\nProduct of x, y, z is : %d", x * y * z);
}
}
}
return 0;
}
Sample Output:
Numbers are: 200, 375, 425 Product of x, y, z is : 31875000
Flowchart:
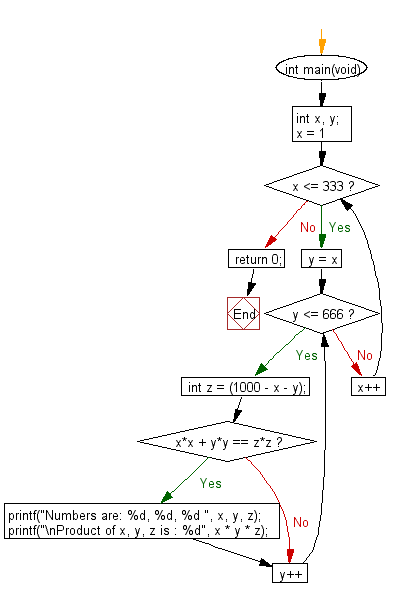
For more Practice: Solve these Related Problems:
- Write a C program to find the first missing positive integer in an unsorted array using constant space.
- Write a C program to rearrange an array so that each index contains its corresponding positive integer, then find the missing one.
- Write a C program to identify the smallest missing positive integer and then print the updated sorted array.
- Write a C program to determine the first missing positive integer using in-place hash mapping techniques.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Numbers with 13 adjacent digits have highest product.
Next C Programming Exercise: Find the sum of all the primes below ten thousand.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.