C Exercises: Find the sum of all the primes below ten thousand
26. Minimum Insertions for Palindrome Variants
A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. A natural number greater than 1 that is not prime is called a composite number. For example, 5 is prime because the only ways of writing it as a product, 1 × 5 or 5 × 1, involve 5 itself. However, 6 is composite because it is the product of two numbers (2 × 3) that are both smaller than 6.
The sum of the primes below 10 is 2 + 3 + 5 + 7 = 17.
Write a C program to find the sum of all prime numbers below ten thousand.
C Code:
#include <stdio.h>
#include <stdlib.h>
int main(void)
{
char *temp;
unsigned i, j;
size_t num = 10000;
unsigned long long sum = 0ULL;
temp = calloc(num, sizeof *temp);
for (i = 2; i < num; i++) {
if (!temp[i]) {
sum += i;
for (j = i*2; j < num; j += i) {
temp[j] = 1;
}
}
}
free(temp);
printf("%llu\n", sum);
return 0;
}
Sample Output:
5736396
Flowchart:
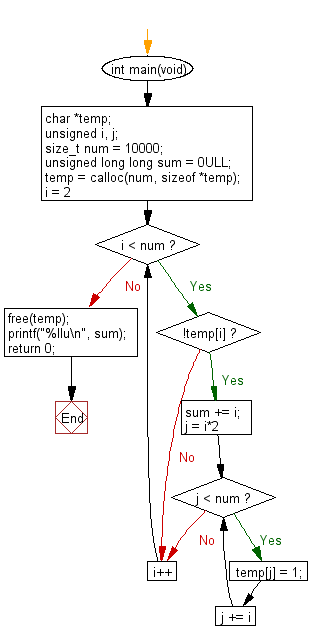
For more Practice: Solve these Related Problems:
- Write a C program to compute the minimum number of insertions required to make a string a palindrome using dynamic programming.
- Write a C program to output the minimum insertions and also reconstruct the resulting palindrome.
- Write a C program to compare the minimum insertions required with the minimum deletions required to form a palindrome.
- Write a C program to find the minimum number of insertions for a string and display the positions where insertions occur.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Find the product xyz.
Next C Programming Exercise: Product of four adjacent numbers in a 20x20 grid.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.