C Exercises: Sort numbers using Bogo Sort method
13. Bogo Sort Variants
Write a C program that sorts numbers using the Bogo Sort method.
In computer science, Bogo Sort is a particularly ineffective sorting algorithm based on the generate and test paradigm. The algorithm successively generates permutations of its input until it finds one that is sorted. It is not useful for sorting but may be used for educational purposes, to contrast it with other more realistic algorithms.
Sample Solution:
Sample C Code:
// BogoSort Implementation
// Source: https://bit.ly/2rcvXK5
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// Function to check if an array is sorted
bool is_sorted(int *a, int n) {
while (--n >= 1) {
if (a[n] < a[n - 1])
return false;
}
return true;
}
// Function to shuffle the elements of an array
void shuffle(int *a, int n) {
int i, t, r;
for (i = 0; i < n; i++) {
t = a[i];
r = rand() % n;
a[i] = a[r];
a[r] = t;
}
}
// BogoSort function to sort an array
void bogosort(int *a, int n) {
while (!is_sorted(a, n))
shuffle(a, n);
}
// Main function
int main() {
int x[] = {1, 10, 9, 7, 3, 0};
int i;
int len = sizeof(x) / sizeof(x[0]);
// Display original array
printf("Original Array:\n");
for (i = 0; i < len; i++)
printf("%d%s", x[i], i == len - 1 ? "\n" : " ");
// Sort the array using BogoSort
printf("\nSorted Array:\n");
bogosort(x, len);
for (i = 0; i < len; i++)
printf("%d ", x[i]);
printf("\n");
return 0;
}
Sample Output:
Original Array: 1 10 9 7 3 0 Sorted Array: 0 1 3 7 9 10
Flowchart:
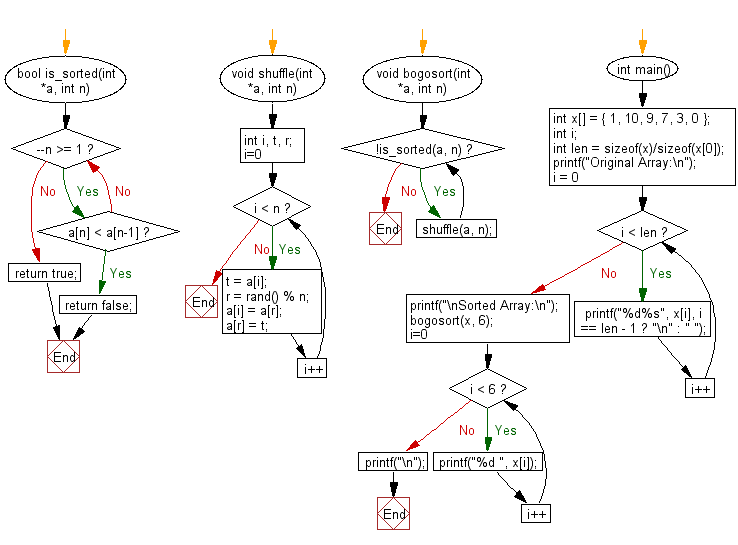
For more Practice: Solve these Related Problems:
- Write a C program to implement bogo sort and count the number of random permutations generated before the array is sorted.
- Write a C program to demonstrate bogo sort on a small dataset and analyze its average-case performance.
- Write a C program to implement a randomized bogo sort that stops after a fixed number of attempts if unsorted.
- Write a C program to compare bogo sort with a conventional sorting algorithm in terms of execution time for educational purposes.
C Programming Code Editor:
Previous: Write a C program that sort numbers using Bead Sort method.
Next: Write a C program that sort numbers using Cocktail Sort method.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.