C Exercises: Bubble sort algorithm
2. Bubble Sort Variants
Write a C program to sort a list of elements using the bubble sort algorithm.
Note: Bubble Sort works by swapping adjacent elements if they are in the wrong order.
Visual presentation - Bubble sort algorithm:
Sample Solution:
Sample C Code:
#include <stdio.h>
// Function to perform bubble sort on an array
void bubble_sort(int *x, int n) {
int i, t, j = n, s = 1;
// Outer loop controls the number of passes
while (s) {
s = 0; // Initialize swap indicator
// Inner loop performs pairwise comparisons and swaps
for (i = 1; i < j; i++) {
if (x[i] < x[i - 1]) {
// Swap elements if they are in the wrong order
t = x[i];
x[i] = x[i - 1];
x[i - 1] = t;
s = 1; // Set swap indicator to 1
}
}
j--; // Reduce the size of the array to ignore the sorted elements
}
}
// Main function
int main() {
int x[] = {15, 56, 12, -21, 1, 659, 3, 83, 51, 3, 135, 0};
int n = sizeof x / sizeof x[0];
int i;
// Print the original array
for (i = 0; i < n; i++)
printf("%d%s", x[i], i == n - 1 ? "\n" : " ");
// Perform bubble sort
bubble_sort(x, n);
// Print the sorted array
for (i = 0; i < n; i++)
printf("%d%s", x[i], i == n - 1 ? "\n" : " ");
return 0;
}
Sample Output:
15 56 12 -21 1 659 3 83 51 3 135 0 -21 0 1 3 3 12 15 51 56 83 135 659
Flowchart:
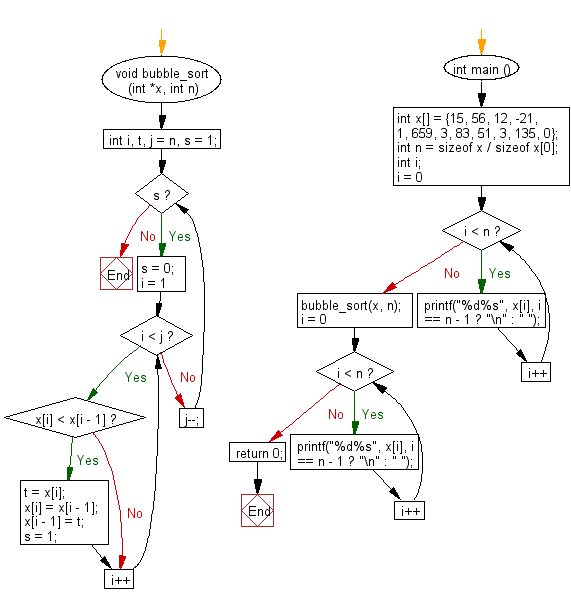
For more Practice: Solve these Related Problems:
- Write a C program to implement bubble sort recursively and count the number of swaps performed.
- Write a C program to optimize bubble sort by stopping early if no swaps occur in a pass and display the iteration count.
- Write a C program to sort an array of strings lexicographically using bubble sort.
- Write a C program to perform bubble sort in descending order and then verify the order using binary search.
C Programming Code Editor:
Previous: Write a C program to sort a list of elements using the selection sort algorithm.
Next: Write a C program to sort a list of elements using the insertion sort algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.