C Exercises: Insertion sort algorithm
3. Insertion Sort Variants
Write a C program to sort a list of elements using the insertion sort algorithm.
Note:
Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time. It is much less efficient on large lists than other algorithms such as quicksort, heapsort, or merge sort.
Visual presentation - Insertion search algorithm:
Sample Solution:
Sample C Code:
#include<stdio.h>
int main() {
int arra[10], i, j, n, array_key;
// Input the number of values in the array
printf("Input no. of values in the array: \n");
scanf("%d", &n);
// Input array values
printf("Input %d array value(s): \n", n);
for (i = 0; i < n; i++)
scanf("%d", &arra[i]);
/* Insertion Sort */
for (i = 1; i < n; i++) {
array_key = arra[i];
j = i - 1;
// Move elements greater than array_key to one position ahead of their current position
while (j >= 0 && arra[j] > array_key) {
arra[j + 1] = arra[j];
j = j - 1;
}
// Insert array_key at its correct position
arra[j + 1] = array_key;
}
// Print the sorted array
printf("Sorted Array: \n");
for (i = 0; i < n; i++)
printf("%d \n", arra[i]);
return 0;
}
Sample Input:
3 12 15 56
Sample Output:
Input no. of values in the array: Input 3 array value(s): Sorted Array: 12 15 56
Flowchart:
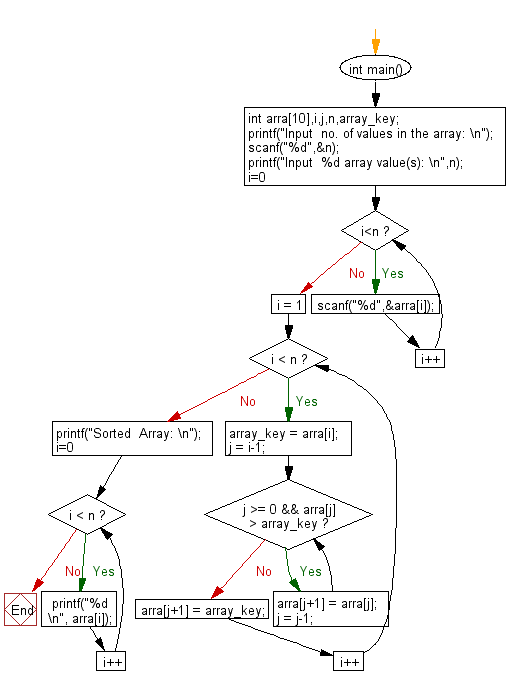
For more Practice: Solve these Related Problems:
- Write a C program to implement insertion sort recursively and measure the recursion depth.
- Write a C program to sort an array using insertion sort while counting the total number of shifts.
- Write a C program to perform insertion sort on an array of floating-point numbers and compute the sorted array's average.
- Write a C program to sort a linked list using insertion sort and then convert it back to an array.
C Programming Code Editor:
Previous: Write a C program to sort a list of elements using the bubble sort algorithm.
Next: Write a C program to sort a list of elements using the merge sort algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.