C Programming: Verify that a string contains valid parentheses
C String: Exercise-36 with Solution
From Wikipedia-
A bracket is either of two tall fore- or back-facing punctuation marks commonly used to isolate a segment of text or data from its surroundings. Typically deployed in symmetric pairs, an individual bracket may be identified as a left or right bracket or, alternatively, an opening bracket or closing bracket, respectively, depending on the directionality of the context.
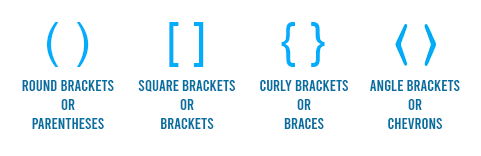
A given string contains the bracket characters '(', ')', '{', '}', '<', ‘>', '[' and ']', Write a C program to check if the string is valid or not. The input string will be valid when open brackets and closed brackets are same type of brackets.
Or
open brackets will be closed in proper order.
Sample Data:
( "<>") -> true
("<>()[]{}") -> true
( "(>") -> false
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
// Function to test if the brackets in the string are balanced
bool test(char *s, int len) {
int ctr = 0, x = 0, y = 0, z = 0;
char *string2;
string2 = (char *)malloc(len); // Allocating memory for a temporary stack
while (*s != 0) {
// Checking for opening brackets and storing them in the temporary stack
if (*s == '(') {
x++;
string2[++ctr] = 1; // Storing the value 1 for '(' in the stack
}
if (*s == '[') {
y++;
string2[++ctr] = 2; // Storing the value 2 for '[' in the stack
}
if (*s == '{') {
z++;
string2[++ctr] = 3; // Storing the value 3 for '{' in the stack
}
// Checking for closing brackets and validating them against the stack content
if (*s == ')') {
if (string2[ctr] == 1) {
x--;
ctr--;
} else {
free(string2); // Freeing the memory allocated for the stack
return false;
}
}
if (*s == ']') {
if (string2[ctr] == 2) {
y--;
ctr--;
} else {
free(string2);
return false;
}
}
if (*s == '}') {
if (string2[ctr] == 3) {
z--;
ctr--;
} else {
free(string2);
return false;
}
}
s++;
}
free(string2); // Freeing the memory allocated for the stack
// Checking if all brackets are balanced
if (x > 0 || y > 0 || z > 0)
return false;
return true;
}
int main() {
char string1[80];
int n, i, x;
printf("Input a string: ");
scanf("%s", string1);
n = strlen(string1);
printf("Check whether brackets in the string are valid or not: %d", test(string1, n));
return 0;
}
Sample Output:
Input a string: <>()[]{} Check bracket in the said string is valid or not? 1
Flowchart :
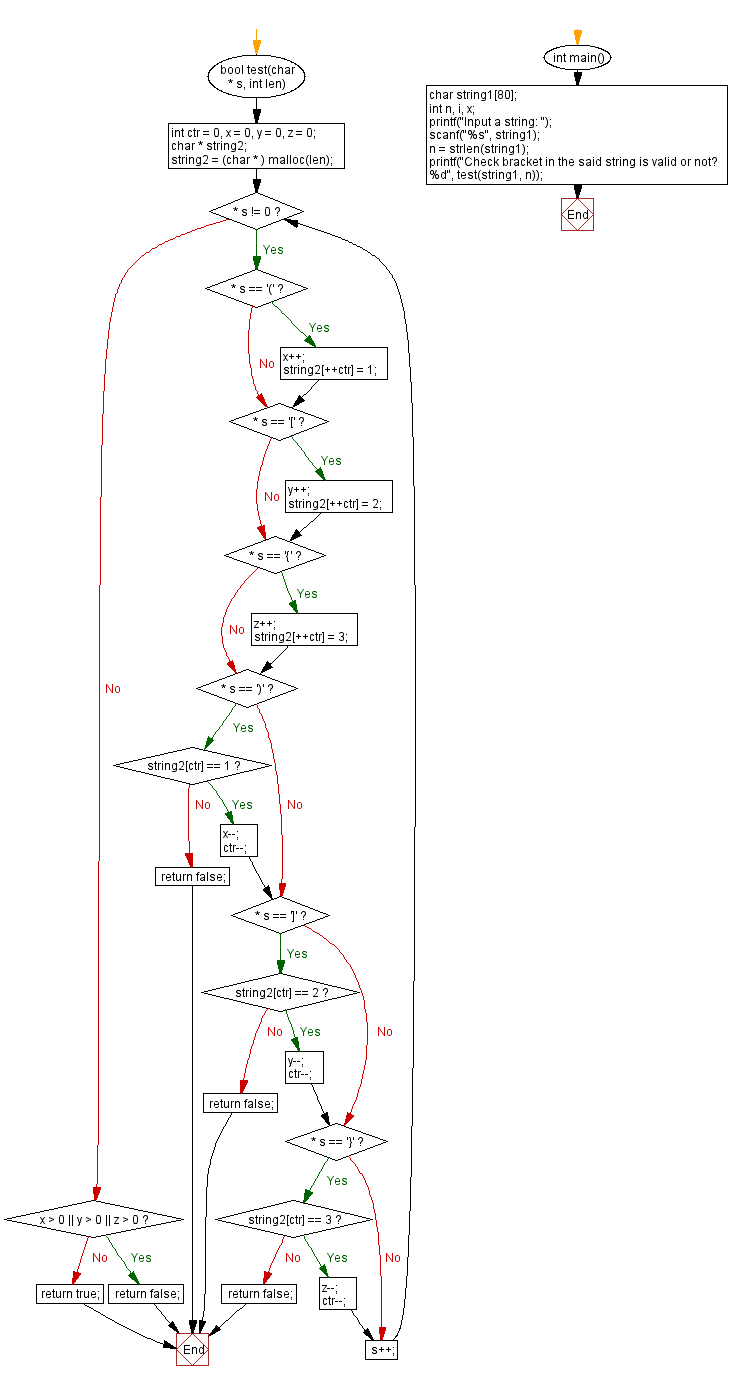
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous C Exercise: Length of the longest substring in a given string.
Next C Exercise: Multiple two positive numbers represent as string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/string/c-string-exercise-36.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics