C Exercises: Convert a string to an unsigned long integer
2. String to Unsigned Long Conversion
Write a C program to convert a string to an unsigned long integer.
Sample Solution:
C Code:
#include<stdio.h>
#include<stdlib.h>
int main ()
{
char buffer[123]; // Define a character array 'buffer' to store user input
unsigned long ul; // Define an unsigned long variable 'ul' to store the converted value
// Print a message asking the user for input
printf ("\nInput an unsigned number: ");
// Read user input from standard input and store it in 'buffer'
fgets (buffer,123,stdin);
// Convert the string in 'buffer' to an unsigned long, using base 0 (auto-detect)
ul = strtoul (buffer,NULL,0);
// Print the converted value
printf ("Output: %lu\n\n",ul);
return 0; // Indicate successful program execution
}
Sample Output:
Input an unsigned number: 25 Output: 25
Flowchart:
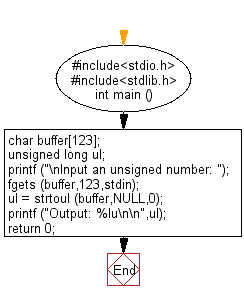
For more Practice: Solve these Related Problems:
- Write a C program to convert a hexadecimal string to an unsigned long integer using strtoul() with error checking.
- Write a C program to parse a string with embedded whitespace into an unsigned long integer and handle invalid input.
- Write a C program to convert a string with a leading '+' sign into an unsigned long integer and detect overflow conditions.
- Write a C program to convert a string to an unsigned long integer and verify that the entire string represents a valid number.
C Programming Code Editor:
Previous: Write a C program which will invoke the command processor to execute a command.
Next: Write a C program to convert a string to a long integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.