C Program: Calculate sum of positive integers using while loop
2. Sum of Positive Integers Until 0
Write a C program that prompts the user to input a series of integers until the user stops entering 0 using a while loop. Calculate and print the sum of all the positive integers entered.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int number; // Variable to store the user input
int sum = 0; // Variable to store the sum of positive integers
printf("Input integers (enter 0 to stop):\n");
// Start a while loop to continue until the user enters 0
while (1) {
printf("Input a number: ");
scanf("%d", &number); // Read the user input
if (number == 0) {
break; // Exit the loop if the user enters 0
}
if (number > 0) {
sum += number; // Add positive numbers to the sum
}
}
// Print the sum of positive integers
printf("Sum of positive integers: %d\n", sum);
return 0; // Indicate successful program execution
}
Sample Output:
Input integers (enter 0 to stop): Input a number: 5 Input a number: 9 Input a number: -3 Input a number: 2 Input a number: 5 Input a number: 6 Input a number: -20 Input a number: 0 Sum of positive integers: 27
Explanation:
Here are key parts of the above code step by step:
- #include <stdio.h>: This line includes the standard input-output library, allowing functions like "printf()" and "scanf()".
- int number;: Declares an integer variable 'number' to store the user input.
- int sum = 0;: Declares an integer variable 'sum' and initializes it to 0. This variable will accumulate the sum of positive integers.
- printf("Input integers (enter 0 to stop):\n");: Prints a message prompting the user to enter integers and telling them to input 0 to stop.
- while (1) {: Starts an infinite "while" loop. The loop will continue until explicitly broken with the "break" statement.
- printf("Input a number: ");: Prompts the user to enter a number.
- scanf("%d", &number);: Reads the user input as an integer and stores it in the variable number.
- if (number == 0) { break; }: Checks if the user entered 0. If true, it breaks out of the infinite loop.
- if (number > 0) { sum += number; }: Checks if the entered number is positive. If true, it adds the number to the sum.
- }: Closes the "while" loop.
- printf("Sum of positive integers: %d\n", sum);: Prints the sum of positive integers entered by the user.
- return 0;: Indicates that the program executed successfully and returns 0 to the operating system.
- }: Closes the "main()" function.
Flowchart:
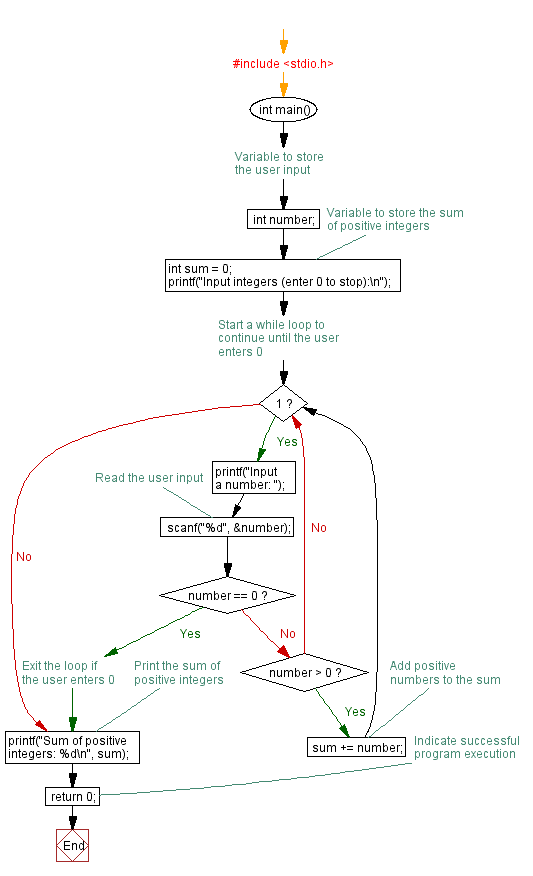
For more Practice: Solve these Related Problems:
- Write a C program to continuously accept integers until 0 is entered and then print the sum of only the positive numbers.
- Write a C program to accept a series of integers until 0 is entered, then compute and display both the sum and count of positive integers.
- Write a C program to accept integers until 0 is entered and output the average of all positive integers entered.
- Write a C program to accept a series of integers until 0 is entered and then print the maximum positive number along with the sum of positives.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: C Program to Print numbers from 0 to 10 and 10 to 0 using While loops
Next: C Program to calculate product of numbers 1 to 5 using while loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.