C Program to calculate product of numbers 1 to 5 using while loop
C While Loop: Exercise-3 with Solution
Write a C program that calculates the product of numbers from 1 to 5 using a while loop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int number = 1; // Initialize the variable to store the current number
int product = 1; // Initialize the variable to store the product
// Start a while loop to iterate from 1 to 5
while (number <= 5) {
// Update the product by multiplying it with the current number
product *= number;
// Print the current number and product in each iteration
printf("Current number: %d, Current product: %d\n", number, product);
// Increment the number for the next iteration
number++;
}
return 0; // Indicate successful program execution
}
Sample Output:
Current number: 1, Current product: 1 Current number: 2, Current product: 2 Current number: 3, Current product: 6 Current number: 4, Current product: 24 Current number: 5, Current product: 120
Explanation:
Here are key parts of the above code step by step:
- #include <stdio.h>: This line includes the standard input-output library, allowing functions like "printf()".
- int number = 1;: Declares an integer variable 'number' and initializes it to 1. This variable is used to store the current number during the loop.
- int product = 1;: Declares an integer variable 'product' and initializes it to 1. This variable is used to store the product of numbers during the loop.
- while (number <= 5) {: Starts a "while" loop that continues as long as 'number' is less than or equal to 5.
- product *= number;: Updates the product by multiplying it with the current value of 'number'.
- printf("Current number: %d, Current product: %d\n", number, product);: Prints the current value of 'number' and the current value of 'product' in each iteration.
- number++;: Increments the value of 'number' for the next iteration.
- }: Closes the "while" loop.
- return 0;: Indicates that the program executed successfully and returns 0 to the operating system.
- }: Closes the "main()" function.
Flowchart:
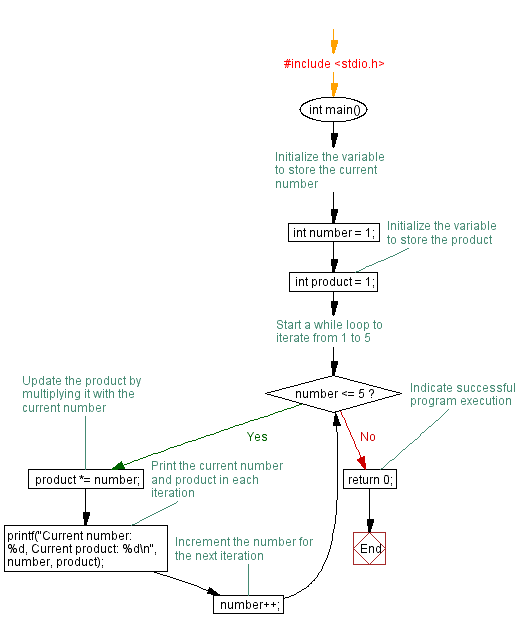
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate sum of positive integers using while loop.
Next: C Program: Detect duplicate numbers using while loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics