C++ Exercises: Find the largest element of a given array of integers
1. Largest Element in Array
Write a C++ program to find the largest element of a given array of integers.
Visual Presentation:
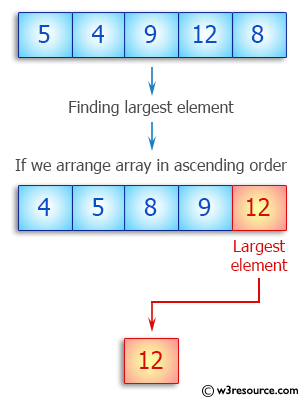
Sample Solution:
C++ Code :
#include <algorithm>
#include<iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
int find_largest(int nums[], int n) { // Function definition for finding the largest element in an array
return *max_element(nums, nums + n); // Return the maximum element in the array using max_element from algorithm library
}
int main() { // Main function where the program execution starts
int nums[] = { // Declaring and initializing an integer array
5,
4,
9,
12,
8
};
int n = sizeof(nums) / sizeof(nums[0]); // Determining the number of elements in the array
cout << "Original array:"; // Output message indicating the original array is being displayed
for (int i=0; i < n; i++) // Loop to display each element of the array
cout << nums[i] <<" "; // Output each element of the array
cout << "\nLargest element of the said array: "<< find_largest(nums, n); // Output message showing the largest element in the array
return 0; // Return statement indicating successful execution and program termination
}
Sample Output:
Original array:5 4 9 12 8 Largest element of the said array: 12
Flowchart:
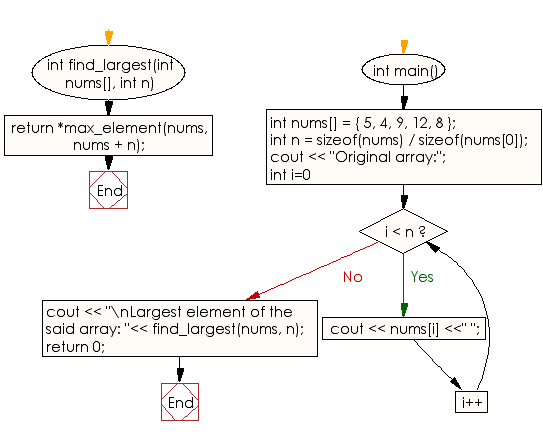
For more Practice: Solve these Related Problems:
- Write a C++ program to determine the largest number in an array using a single loop and conditional statements.
- Write a C++ program that finds the maximum element in an unsorted array by iterating through the array and updating a maximum variable.
- Write a C++ program to compute the largest element in an array using the std::max_element algorithm without explicit loops.
- Write a C++ program that reads an array from input and prints the largest value, ensuring to handle negative numbers correctly.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: C++ Array Exercises Home
Next: Write a C++ program to find the largest three elements in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.