C++ Exercises: Sort an array of distinct elements according to absolute difference of array elements and with a given value
C++ Array: Exercise-17 with Solution
Write a C++ program to sort (in descending order) an array of distinct elements according to the absolute difference of array elements and with a given value.
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
#include <map> // Header file for the map and multimap containers
using namespace std; // Using the standard namespace
// Function to rearrange array elements based on their absolute difference from a given number 'x'
void rearrange_array_elements(int nums[], int n, int x)
{
multimap<int, int> m; // Create a multimap to store absolute differences as keys and array elements as values
// Insert elements into the multimap with their absolute differences from 'x' as keys
for (int i = 0; i < n; i++)
m.insert(make_pair(abs(x - nums[i]), nums[i])); // Use 'make_pair' to create a pair of (absolute difference, array element)
int i = 0; // Initialize an index variable for accessing array elements after rearrangement
// Traverse the multimap and rearrange the array based on sorted absolute differences
for (auto t = m.begin(); t != m.end(); t++)
nums[i++] = (*t).second; // Assign the elements sorted by absolute difference back to the original array
}
// Main function
int main()
{
int nums[] = {0, 9, 7, 2, 12, 11, 20}; // Declaration and initialization of an integer array
int n = sizeof(nums) / sizeof(nums[0]); // Calculate the number of elements in the array
cout << "Original array: ";
int x = 12; // Given number 'x'
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the original array
rearrange_array_elements(nums, n, x); // Rearrange the array elements based on their absolute differences from 'x'
cout << "\nArray elements after rearrange: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the modified array after rearrangement
return 0;
}
Sample Output:
Original array: 0 9 7 2 12 11 20 Array elements after rearrange: 12 11 9 7 20 2 0
Flowchart:
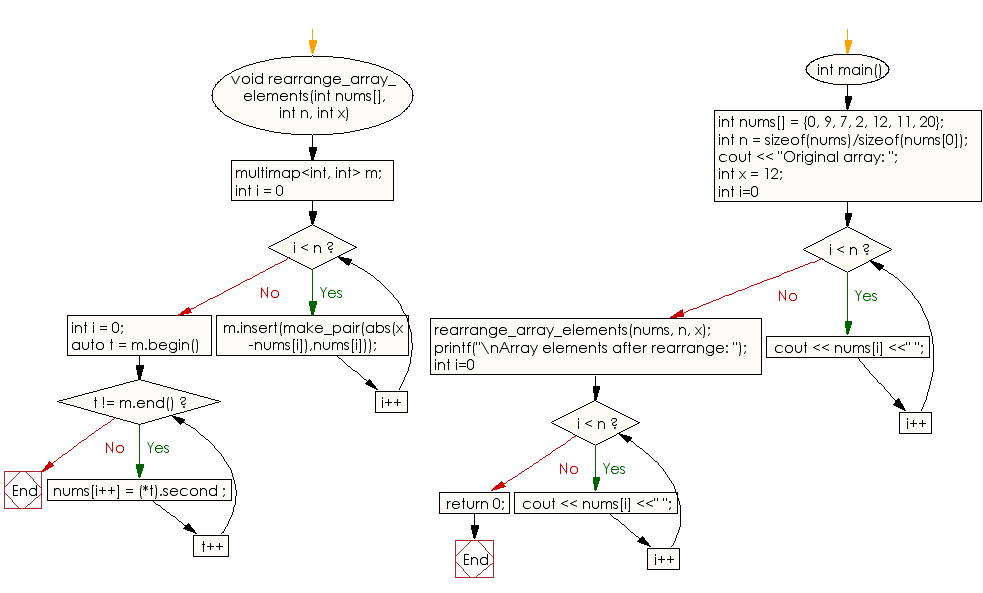
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to sort a given array of 0s, 1s and 2s. In the final array put all 0s first, then all 1s and all 2s in last.
Next: Write a C++ program to move all negative elements of an array of integers to the end of the array without changing the order of positive element and negative element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/array/cpp-array-exercise-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics