C++ Exercises: Find a number which occurs odd number of times of a given array of positive integers
19. Find Element Occurring Odd Number of Times
Write a C++ program to find a number that occurs an odd number of times in a given array of positive integers. In the array, all numbers occur an even number of times.
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to find the element occurring an odd number of times in an array
int getOddOccurrence(int nums[], int n)
{
for (int i = 0; i < n; i++) { // Loop through each element in the array
int ctr = 0; // Counter to count occurrences of nums[i]
for (int j = 0; j < n; j++) // Loop to count occurrences of nums[i] in the array
{
if (nums[i] == nums[j]) // If the current element matches nums[i]
ctr++; // Increment the counter
}
if (ctr % 2 != 0) // If the counter is odd
return nums[i]; // Return the element occurring odd times
}
return -1; // Return -1 if no element occurs an odd number of times
}
int main()
{
int nums[] = {5, 7, 8, 8, 5, 8, 7, 7}; // Declaration and initialization of an integer array
int n = sizeof(nums)/sizeof(nums[0]); // Calculate the number of elements in the array
cout << "Original array: ";
for (int i=0; i < n; i++)
cout << nums[i] <<" "; // Output each element of the original array
cout << "\nNumber which occurs odd number of times: " << getOddOccurrence(nums, n); // Call function to find the element occurring odd times
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Original array: 5 7 8 8 5 8 7 7 Number which occurs odd number of times: 7
Flowchart:
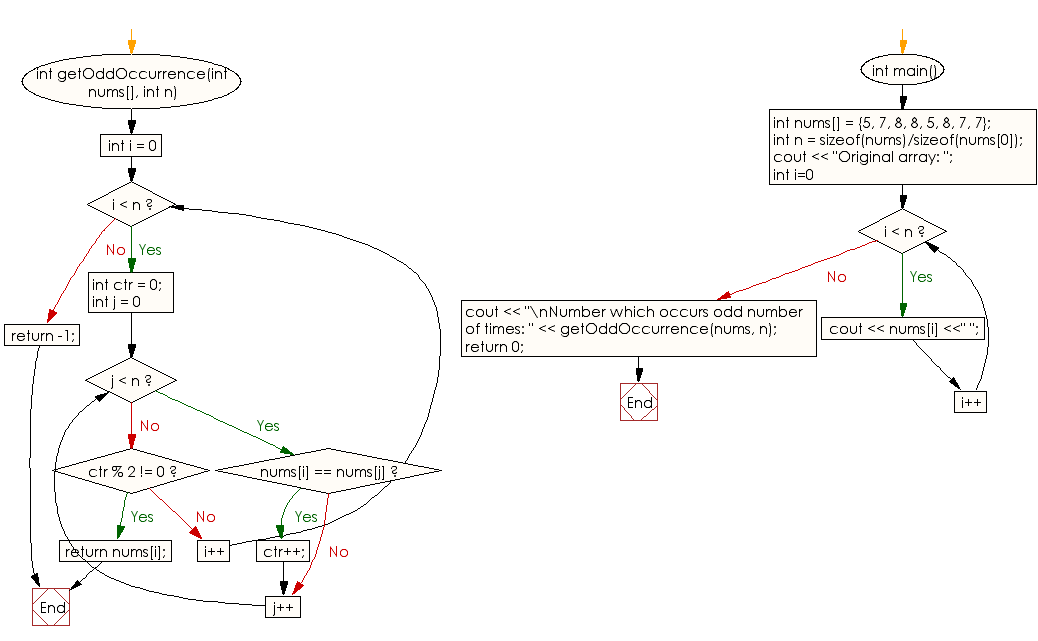
For more Practice: Solve these Related Problems:
- Write a C++ program to identify the element that occurs an odd number of times in an array where all other elements occur even times using bitwise XOR.
- Write a C++ program that reads an array and outputs the element with odd frequency by applying the XOR property over the entire array.
- Write a C++ program to determine and print the unique element that appears an odd number of times in a given array.
- Write a C++ program that processes an array of integers and uses the XOR operation to find the odd-occurring element.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to move all negative elements of an array of integers to the end of the array without changing the order of positive element and negative element.
Next: Write a C++ program to count the number of occurrences of given number in a sorted array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.