C++ Exercises: Find the second smallest elements in a given array of integers
5. Second Smallest Element in Array
Write a C++ program to find the second smallest elements in a given array of integers.
Visual Presentation:
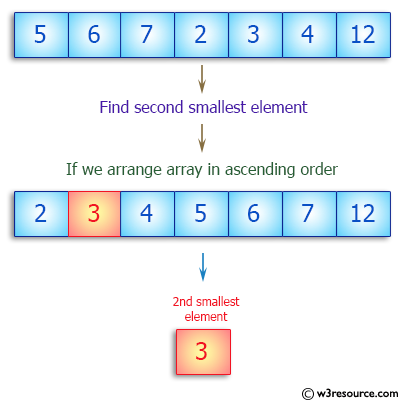
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to find the second smallest element in an array
int find_Second_Smallest(int array_num[], int n) {
int smallest_num, second_smallest_num; // Declaring variables to store smallest and second smallest numbers
// Determining initial smallest and second smallest numbers from the first two elements of the array
if (array_num[0] < array_num[1]) {
smallest_num = array_num[0];
second_smallest_num = array_num[1];
} else {
smallest_num = array_num[1];
second_smallest_num = array_num[0];
}
// Loop to find the second smallest number in the array
for (int i = 0; i < n; i++) {
// If current element is smaller than the smallest number
if (smallest_num > array_num[i]) {
second_smallest_num = smallest_num; // Assign the smallest number to second smallest
smallest_num = array_num[i]; // Update the smallest number
}
// If current element is greater than smallest but smaller than second smallest
else if (array_num[i] < second_smallest_num && array_num[i] > smallest_num) {
second_smallest_num = array_num[i]; // Update the second smallest number
}
}
return second_smallest_num; // Return the second smallest number found
}
int main() {
int n = 7; // Number of elements in the array
int array_num[7] = {5, 6, 7, 2, 3, 4, 12}; // Declaration and initialization of an integer array
int s = sizeof(array_num) / sizeof(array_num[0]); // Determining the size of the array
cout << "Original array: "; // Output message indicating the original array is being displayed
for (int i=0; i < s; i++)
cout << array_num[i] <<" "; // Output each element of the array
int second_smallest_num = find_Second_Smallest(array_num, n); // Finding the second smallest number in the array
cout<<"\nSecond smallest number: "<<second_smallest_num; // Output the second smallest number found
return 0;
}
Sample Output:
Original array: 5 6 7 2 3 4 12 Second smallest number: 3
Flowchart:
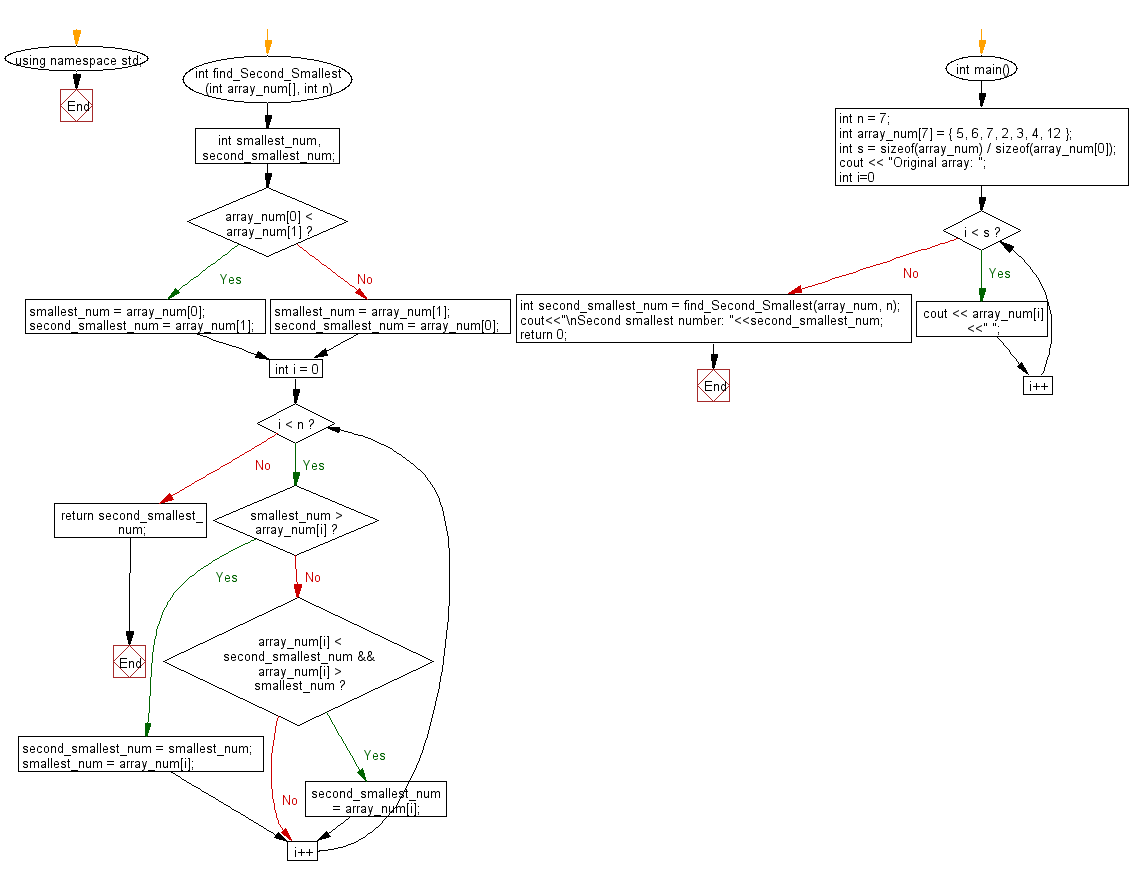
For more Practice: Solve these Related Problems:
- Write a C++ program to determine the second smallest element in an array by tracking the smallest and second smallest values.
- Write a C++ program that sorts the array in ascending order and then selects the second unique smallest number.
- Write a C++ program to scan an array and update two variables to capture the minimum and second minimum values simultaneously.
- Write a C++ program that reads an array and prints the second smallest element while handling duplicate minimums appropriately.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find k largest elements in a given array of integers.
Next: Write a C++ program to find all elements in array of integers which have at-least two greater elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.