C++ Exercises: Check if there are two values 15, 15 adjacent to each other in a given array of integers
Check if Two Adjacent '15's Exist in Array
Write a C++ program to check if there are two values 15, 15 adjacent to each other in a given array (length should be at least 2) of integers. Return true otherwise false.
Test Data:
({ 5, 5, 1, 15, 15 }) -> 1
({ 15, 2, 3, 4, 15 }) -> 0
({ 3, 3, 15, 15, 5, 5}) -> 1
({ 1, 5, 15, 7, 8, 15})-> 0
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function to check if there are adjacent elements equal to 15 in the array
static bool test(int numbers[], int arr_length)
{
for (int i = 0; i < arr_length - 1; i++)
{
if (numbers[i + 1] == numbers[i] && numbers[i] == 15) return true; // If two adjacent elements are both equal to 15, return true
}
return false; // Return false if no adjacent elements are equal to 15
}
// Main function
int main()
{
// Different test cases with arrays of integers
int nums1[] = {5, 5, 1, 15, 15};
int arr_length = sizeof(nums1) / sizeof(nums1[0]);
cout << test(nums1, arr_length) << endl;
int nums2[] = {15, 2, 3, 4, 15};
arr_length = sizeof(nums2) / sizeof(nums2[0]);
cout << test(nums2, arr_length) << endl;
int nums3[] = {3, 3, 15, 15, 5, 5};
arr_length = sizeof(nums3) / sizeof(nums3[0]);
cout << test(nums3, arr_length) << endl;
int nums4[] = {1, 5, 15, 7, 8, 15};
arr_length = sizeof(nums4) / sizeof(nums4[0]);
cout << test(nums4, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 0 1 0
Flowchart:
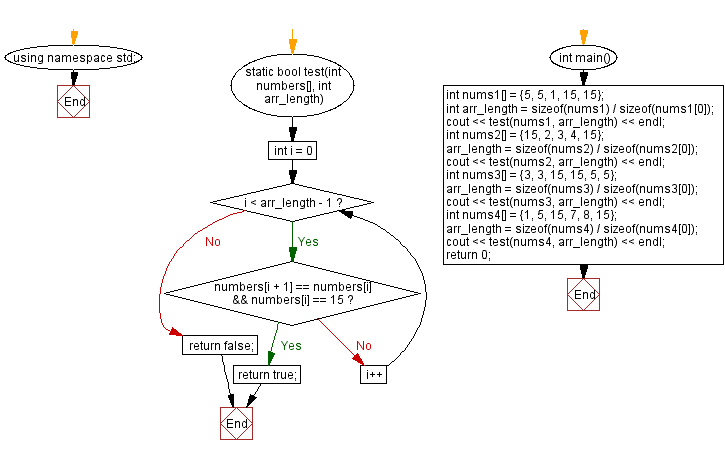
For more Practice: Solve these Related Problems:
- Write a C++ program to check if an array contains two consecutive occurrences of the number 15 and output true if found.
- Write a C++ program that reads an array and returns true if a pair of adjacent 15's exists, otherwise false.
- Write a C++ program to iterate through an array and print 1 if it detects a consecutive pair of 15's, else 0.
- Write a C++ program to examine a 2-or-more element array and determine whether 15 appears in two consecutive positions.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Check if the value of each element is equal or greater than the value of previous element of a given array of integers.
Next: Find the largest average value between the first and second halves of a given array of integers.
What is the difficulty level of this exercise?